Code Optimization Techniques: Efficient Programming
Programming is not just about writing code; it’s about writing efficient and optimized code that can make a significant difference in the performance of software applications. In this article, we’ll delve into the world of code optimization techniques, exploring the various strategies developers use to enhance the speed, efficiency, and overall performance of their code.
Contents
1. Introduction
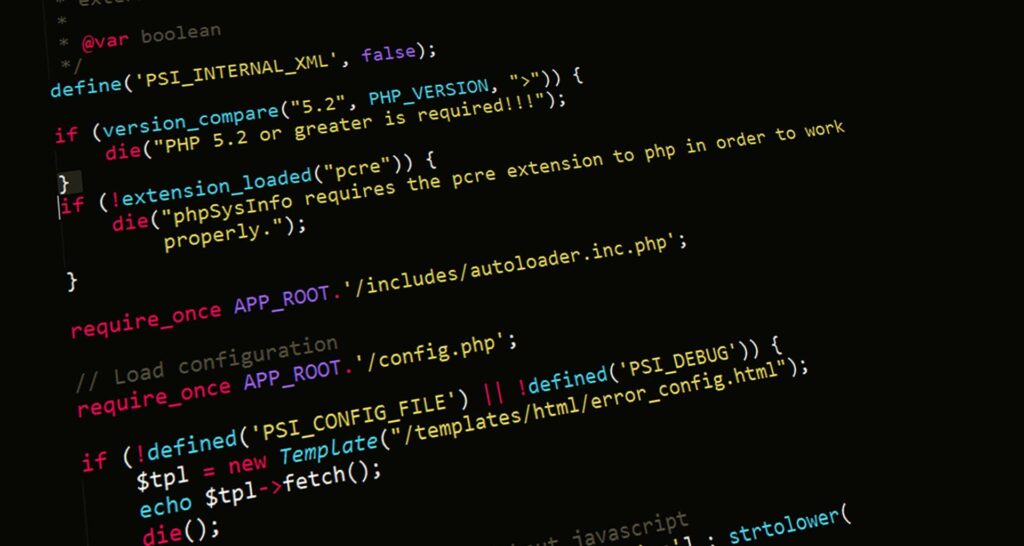
Defining Code Optimization
Code optimization is the process of refining and improving the efficiency of a computer program. It involves making the code run faster, use fewer system resources, and ultimately deliver a better user experience. This optimization process is crucial, especially as software complexity continues to grow.
Importance in Software Development
Efficient code is not just a luxury; it’s a necessity. In a world where users demand faster applications and seamless experiences, optimizing code becomes a fundamental aspect of software development. It not only improves user satisfaction but also contributes to overall system stability.
2. Understanding the Basics
What is Code Efficiency?
Code efficiency refers to how well a program utilizes system resources to perform its intended functions. An efficient code executes tasks quickly, minimizes memory usage, and reduces the overall computational load on a system.
The Impact of Inefficient Code on Performance
Inefficient code can lead to slow program execution, increased memory consumption, and a negative impact on the user experience. Developers often face the challenge of balancing functionality with performance to create applications that are both feature-rich and fast.
3. Common Code Optimization Techniques
Variable Optimization
Optimizing variable usage involves minimizing the number of variables and using appropriate data types. This not only saves memory but also improves the speed of variable manipulation.
- Use Meaningful Variable Names: Instead of generic names like
total
ortemp
, use names that convey the purpose of the variable. For example, usetotal_price
instead of justtotal
. - Avoid Unnecessary Variables: If a variable is used only once or its value doesn’t change, consider eliminating it and directly using the value in the calculation.
- Choose Appropriate Data Types: Opt for data types that match the nature of the data. Using integers instead of floats when decimal precision is unnecessary can save memory.
- Minimize Redundant Operations: Avoid unnecessary operations within loops. For instance, calculate values outside the loop if they remain constant throughout the iteration.
Loop Optimization
Loops are integral to programming, and optimizing them can significantly impact performance. Techniques like loop unrolling and loop fusion help streamline code execution.
Inefficient Loop
for (int i = 0; i < arr.Length; i++)
{
result[i] = arr[i] * 2; // Performing a simple operation on each element
}
This loop is inefficient because it calculates arr.Length
in every iteration. Storing the length in a separate variable outside the loop can improve performance.
Optimized Loop
int length = arr.Length; // Store the array length outside the loop
for (int i = 0; i < length; i++)
{
result[i] = arr[i] * 2; // Performing a simple operation on each element
}
By storing the array length outside the loop, we eliminate the redundant calculation in each iteration, resulting in a more optimized loop. This optimization is particularly noticeable in larger loops or when the array length doesn’t change during the loop execution. Always remember that micro-optimizations might have a minimal impact in simple cases but can be crucial in performance-critical scenarios.
Algorithmic Improvements
Optimizing algorithms can lead to substantial performance gains. Evaluating and choosing the most efficient algorithm for a specific task is a critical aspect of code optimization techniques.
4. Memory Management Techniques
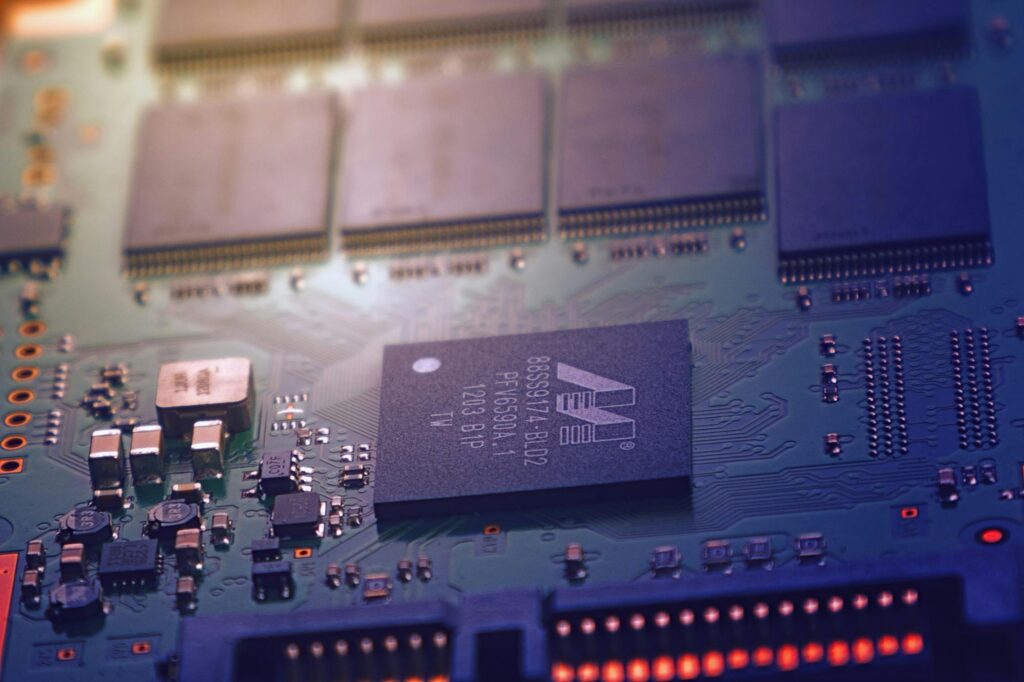
Dynamic Memory Allocation
Efficient memory allocation and deallocation are essential for preventing memory leaks and enhancing overall system stability. Techniques like pooling and smart pointers can be employed for better memory management.
Data Structure Optimization
Choosing the right data structures for specific tasks can greatly influence code efficiency. Optimal data structures reduce the time complexity of operations, leading to faster program execution.
5. Utilizing Compiler Optimizations
Compiler Flags and Settings
Modern compilers offer a range of optimization flags and settings that developers can leverage to enhance code performance. Understanding these options is key to unleashing the full potential of a compiler.
Inlining and Outlining
Inlining involves incorporating the code of small functions directly into the calling function, reducing function call overhead. Outlining, on the other hand, focuses on extracting specific code into separate functions for better readability and maintainability.
6. Parallelization and Concurrency
Multithreading and Parallel Processing
Breaking down tasks into smaller, parallelizable units can lead to significant speed improvements. Multithreading and parallel processing distribute the workload across multiple threads or processors, maximizing system resources.
Asynchronous Programming
Asynchronous programming allows non-blocking execution of tasks, enabling a program to perform other operations while waiting for certain tasks to complete. This enhances overall program responsiveness.
7. Platform-Specific Optimization
CPU Architecture Considerations
Understanding the target CPU architecture is crucial for optimizing code for specific platforms. Utilizing architecture-specific instructions and features can result in substantial performance gains.
Utilizing Hardware Acceleration
Leveraging hardware acceleration, such as GPU computing, can offload certain computations from the CPU, leading to improved overall system performance.
8. Testing and Profiling
Importance of Benchmarking
Benchmarking involves comparing the performance of different code implementations. Rigorous benchmarking helps identify bottlenecks and areas for improvement.
Profiling Tools for Code Analysis
Profiling tools provide insights into code execution, highlighting areas that consume the most resources. Developers can then focus on optimizing these critical sections.
9. Best Practices for Code Optimization Techniques
Maintainability vs. Optimization
Finding the right balance between code maintainability and optimization is crucial. Optimized code should not sacrifice readability, as maintainability is equally important for long-term success.
Balancing Trade-offs
Code optimization often involves trade-offs. Developers must weigh the benefits of optimization against the potential downsides, such as increased complexity or reduced code clarity.
10. Future Trends in Code Optimization
Machine Learning for Code Improvement
The integration of machine learning algorithms in code optimization is an emerging trend. AI-driven tools can analyze code patterns and suggest optimizations, ushering in a new era of automated efficiency.
Integration of AI in Programming
Beyond code optimization, artificial intelligence is increasingly influencing the entire software development lifecycle. From automated testing to code generation, AI is becoming a valuable ally for developers seeking to enhance their productivity.
Conclusion
In the ever-evolving landscape of software development, code optimization remains a critical skill for programmers. From the basics of understanding code efficiency to advanced techniques like parallelization and platform-specific optimizations, developers have a plethora of tools at their disposal. Striking the right balance between functionality, maintainability, and performance is the key to creating robust and efficient software.
Frequently Asked Questions (FAQs)
- Is code optimization techniques only about making code run faster? Code optimization techniques encompasses various aspects, including improving speed, reducing memory usage, and enhancing overall efficiency. It goes beyond just making code run faster.
- How do I choose the right algorithm for optimization? Selecting the right algorithm involves considering factors such as the nature of the task, input size, and desired performance. Profiling and benchmarking can aid in making informed decisions.
- Are there risks associated with aggressive code optimization? Aggressive optimization may lead to increased code complexity and reduced maintainability. It’s essential to balance optimization efforts with the need for clear and understandable code.
- Can code optimization be automated using AI? Yes, the integration of machine learning in code optimization is an emerging trend. AI tools can analyze code patterns and suggest optimizations, streamlining the optimization process.
- Is code optimization only relevant for large-scale applications? Code optimization is relevant for applications of all sizes. Even small improvements in code efficiency can have a noticeable impact on the performance of software.