Transact-SQL (T-SQL): Comprehensive Guide
Welcome to the Writing Transact-SQL Statements tutorial. T-SQL (Transact-SQL) is an extension of SQL language. This tutorial covers the fundamental concepts of T-SQL. Each topic is explained using examples for easy understanding.
Contents
Overview
Transact-SQL (T-SQL) is Microsoft’s and Sybase’s proprietary extension to the SQL (Structured Query Language) used to interact with relational databases.
In 1970’s the product called “SEQUEL”, Structured English QUEry Language, developed by IBM and later “SEQUEL” was renamed to “SQL” which stands for Structured Query Language.
In 1986, SQL was approved by ANSI (American national Standards Institute) and in 1987, it was approved by ISO (International Standards Organization).
Importance of T-SQL in Database Management In the realm of database management, T-SQL plays a crucial role in facilitating various tasks such as retrieving data, modifying database objects, and implementing business logic within database applications. Its rich set of features empowers developers to write complex queries, automate processes, and ensure the integrity and security of the data stored in SQL Server databases.
Basic Concepts of Transact-SQL
Data Types in T-SQL T-SQL supports a wide range of data types, including integers, strings, dates, and binary data. Understanding and appropriately choosing data types is essential for efficient storage and manipulation of data in SQL Server databases.
Variables and Data Manipulation Variables in T-SQL enable storage and manipulation of values within scripts and stored procedures. They can hold various data types and are useful for dynamic query generation, iterative processing, and temporary storage of intermediate results.
Transact-SQL Syntax
Understanding SQL Statements T-SQL syntax follows the standard SQL conventions for writing statements such as SELECT, INSERT, UPDATE, DELETE, and others. These statements form the building blocks of database interactions, allowing users to retrieve, modify, and manage data stored in SQL Server databases.
Writing Queries in T-SQL Queries in T-SQL are constructed using SQL statements to retrieve data from one or more tables based on specified criteria. The SELECT statement is commonly used for this purpose, along with clauses like WHERE, ORDER BY, and GROUP BY to filter, sort, and group the results as needed.
1. Data Types:
T-SQL supports various data types to store different kinds of information. Here’s an example creating a table named Customers
to store customer details:
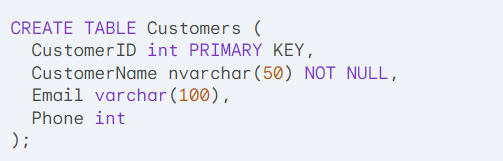
In this example:
int
stores integer values (CustomerID and Phone).nvarchar(50)
stores character strings with a maximum length of 50 characters (CustomerName).varchar(100)
stores character strings with a maximum length of 100 characters (Email) but can be shorter.NOT NULL
specifies that the column cannot contain null values.PRIMARY KEY
defines a unique identifier for each customer (CustomerID).
2. Control Flow Statements:
T-SQL allows using control flow statements like IF
, ELSE
, and WHILE
loops for more complex operations. Here’s a basic example:
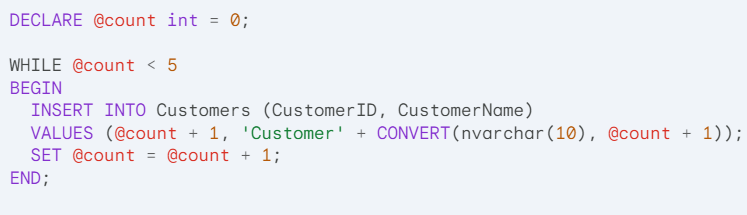
Data Retrieval with Transact-SQL
SELECT Statement and Its Usage The SELECT statement is the primary means of retrieving data from SQL Server tables. It allows users to specify the columns to be retrieved and apply filtering criteria to narrow down the result set. Additionally, it supports various functions and expressions for manipulating the returned data.
Filtering and Sorting Data T-SQL provides powerful mechanisms for filtering data using the WHERE clause, which allows users to specify conditions that must be met for rows to be included in the result set. Sorting of data can be achieved using the ORDER BY clause, which arranges the rows based on one or more columns in ascending or descending order.
Data Modification with Transact-SQL
INSERT, UPDATE, and DELETE Statements T-SQL enables users to modify data in SQL Server tables using the INSERT, UPDATE, and DELETE statements. These statements allow for adding new records, modifying existing ones, and removing unwanted data from tables, respectively.
Managing Data in Tables In addition to basic data modification operations, T-SQL provides features for managing tables, such as creating, altering, and dropping tables. These operations are essential for designing and maintaining the structure of a database schema.
T-SQL Functions
Scalar Functions Scalar functions in T-SQL operate on a single value and return a single value. They can be used in various contexts, such as data manipulation, string manipulation, date and time calculations, and mathematical operations.
Aggregate Functions Aggregate functions in Transact-SQL perform calculations across multiple rows and return a single result. Common aggregate functions include SUM, AVG, COUNT, MIN, and MAX, which are used for summarizing and analyzing data in SQL Server databases.
Control Flow in T-SQL
IF…ELSE Statements IF…ELSE statements in T-SQL provide conditional execution of code based on specified conditions. They are commonly used to implement branching logic within Transact-SQL scripts and stored procedures.
CASE Expressions CASE expressions in Transact-SQL allow for conditional evaluation of expressions. They provide a flexible way to perform conditional logic and return different values based on specified criteria.
Joins and Subqueries in Transact-SQL
Understanding Joins Joins in Transact-SQL are used to combine data from multiple tables based on related columns. Common types of joins include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN, each serving different purposes in retrieving data from relational databases.
Using Subqueries for Complex Queries Subqueries in T-SQL are queries nested within other queries, allowing for the execution of complex logic and data manipulation. They can be used to filter, sort, and aggregate data before being used in the outer query, providing a powerful tool for building sophisticated queries.
Transactions and Error Handling
ACID Properties of Transactions Transactions in T-SQL ensure the ACID properties: Atomicity, Consistency, Isolation, and Durability. They enable users to group multiple database operations into a single unit of work, ensuring data integrity and reliability.
Error Handling in T-SQL T-SQL provides mechanisms for handling errors that may occur during the execution of database operations. This includes try…catch blocks for capturing and handling exceptions, as well as functions and system views for retrieving information about errors.
Stored Procedures and Functions
Creating and Executing Stored Procedures Stored procedures in T-SQL are precompiled sets of one or more SQL statements stored in the database. They offer advantages such as improved performance, code reusability, and enhanced security. Stored procedures can be executed from client applications or other T-SQL scripts.
Defining and Using User-Defined Functions User-defined functions (UDFs) in T-SQL allow developers to encapsulate reusable logic for performing specific tasks. They can be scalar functions, table-valued functions, or inline table-valued functions, providing flexibility in how data is processed and returned.
Indexing and Performance Optimization
Importance of Indexes in T-SQL Indexes in T-SQL are data structures that improve the speed of data retrieval operations by enabling quick access to specific rows within a table. Proper indexing is essential for optimizing query performance and reducing the time taken to execute queries.
Techniques for Improving Query Performance In addition to indexing, various techniques can be employed to enhance the performance of T-SQL queries. These include optimizing query execution plans, minimizing the use of costly operations, and leveraging features like query hints and query optimization tools.
Security in Transact-SQL
Managing Permissions Security in T-SQL revolves around controlling access to database objects and operations. This involves granting appropriate permissions to users and roles, implementing authentication mechanisms, and auditing user activities to ensure compliance with security policies.
Protecting Sensitive Data T-SQL provides mechanisms for encrypting sensitive data stored in SQL Server databases, thereby safeguarding it from unauthorized access. Techniques such as transparent data encryption (TDE), cell-level encryption, and data masking can be used to protect data at rest and in transit.
Advanced Transact-SQL Features
Common Table Expressions (CTEs) CTEs in T-SQL provide a way to define temporary result sets within a query. They improve readability and maintainability by breaking down complex queries into smaller, more manageable parts, and can be used recursively to perform hierarchical or recursive operations.
Window Functions Window functions in T-SQL perform calculations across a set of rows related to the current row, without modifying the result set. They are particularly useful for analytical queries that require comparing or aggregating data within a specified window or partition.
Integration with Other Technologies
T-SQL and .NET T-SQL can be seamlessly integrated with the .NET framework, allowing developers to leverage the power of both platforms in building database-driven applications. This integration enables functionalities such as executing T-SQL scripts from .NET code, accessing SQL Server data in .NET applications, and implementing business logic using CLR (Common Language Runtime) objects.
T-SQL and PowerShell PowerShell is a powerful scripting language and automation framework developed by Microsoft. T-SQL can be invoked from PowerShell scripts using the SQL Server PowerShell module, enabling administrators to automate database management tasks, perform routine maintenance operations, and interact with SQL Server instances programmatically.
Best Practices for Transact-SQL Development
Writing Efficient and Maintainable Code Adhering to best practices is essential for developing T-SQL code that is efficient, robust, and easy to maintain. This includes following naming conventions, using comments to document code, avoiding deprecated features, and optimizing queries for performance.
Continuous Learning and Improvement The field of T-SQL and database management is constantly evolving, with new features, technologies, and best practices emerging over time. Continuous learning and staying updated with the latest developments are essential for T-SQL professionals to enhance their skills, adapt to changes, and deliver high-quality solutions.
Conclusion
Transact-SQL (T-SQL) is a versatile and powerful language for interacting with SQL Server databases. By mastering T-SQL fundamentals and advanced features, developers, administrators, and analysts can effectively manage data, optimize query performance, and build robust database applications. With its broad range of capabilities and integration options, T-SQL remains a cornerstone of modern database management.
FAQs (Frequently Asked Questions)
1. What is the difference between SQL and T-SQL? SQL (Structured Query Language) is a standard language for managing relational databases, while T-SQL (Transact-SQL) is a proprietary extension developed by Microsoft specifically for use with SQL Server.
2. Can T-SQL be used with other database management systems besides SQL Server? While T-SQL is primarily associated with SQL Server, some aspects of its syntax and functionality may be compatible with other database systems that support SQL.
3. How can I improve the performance of T-SQL queries? Performance optimization techniques for T-SQL queries include proper indexing, minimizing data retrieval, optimizing query execution plans, and leveraging caching mechanisms.
4. Are there any security considerations when using T-SQL? Yes, security in T-SQL involves managing permissions, protecting sensitive data, implementing encryption mechanisms, and auditing user activities to ensure compliance with security policies.
5. What resources are available for learning T-SQL? There are numerous resources available for learning T-SQL, including online tutorials, books, documentation from Microsoft, and community forums where users can seek help and advice from experienced professionals.
This article was crafted to provide comprehensive insights into Transact-SQL (T-SQL) and its various aspects. For further inquiries or assistance, feel free to reach out.