Contents
Domain-Driven Design (DDD) has become a cornerstone in modern software development, and understanding its fundamental concepts is crucial for building robust and scalable applications. In this article, we will delve into a specific aspect of DDD Entities and explore their significance, characteristics, creation, and implementation in real-world projects.
What is DDD?
Before we dive into entities, let’s establish a foundation by defining Domain-Driven Design. DDD is an approach to software development that emphasizes the importance of understanding and modeling the domain of the software to ensure its success. It’s not just about writing code; it’s about creating a shared understanding between domain experts and developers to build effective solutions.
Understanding Entities in DDD
In the context of DDD, an entity is a distinct and identifiable concept within the domain that is crucial for the business. Unlike value objects, which are defined by their attributes, entities are defined by a unique identity that runs through time and different states. For instance, in an e-commerce system, a product can be considered an entity with a unique ID, even if its price or description changes.
Key Features of DDD Entities
Entities in DDD exhibit certain key features that set them apart. Immutability is a crucial aspect – once an entity is created, its identity remains unchanged. Additionally, entities often have relationships with other entities, forming the building blocks of the domain model. Understanding and leveraging these features is essential for effective entity design.
Creating Domain-Driven Design Entities
Defining DDD entities involves a thoughtful process. Start by identifying the entities in the domain, focusing on their unique characteristics and the role they play in business processes. Consider the relationships between entities, ensuring a clear and cohesive representation of the domain. Examples of well-defined DDD entities include customer, order, or invoice in various business applications.
Let’s consider an example of DDD entities in the context of an e-commerce application. In this scenario, we’ll focus on two primary entities: Product
and Order
.
Product Entity:
- Attributes:
productId
(Unique identifier)productName
description
price
quantityInStock
- Characteristics:
- Immutable once created (productId remains constant)
- Represents a distinct item in the e-commerce catalog
- Encapsulates business rules, such as minimum stock levels
- Relationships:
- May have relationships with other entities, like
Category
orManufacturer
- Associated with multiple
OrderItem
instances when included in an order
- May have relationships with other entities, like
public class Product {
private final UUID productId;
private final String productName;
private final String description;
private final BigDecimal price;
private int quantityInStock;
// Constructor, getters, and business logic methods
}
Order Entity:
- Attributes:
orderId
(Unique identifier)customer
(Customer placing the order)orderDate
status
(e.g., Pending, Shipped, Delivered)
- Characteristics:
- Immutable once created (orderId remains constant)
- Represents a customer’s purchase request
- Encapsulates business rules, such as order status transitions
- Relationships:
- Contains multiple
OrderItem
instances representing products in the order - Connected to a
Customer
entity
- Contains multiple
public class Order {
private final UUID orderId;
private final Customer customer;
private final LocalDateTime orderDate;
private OrderStatus status;
private final List<OrderItem> orderItems;
// Constructor, getters, and business logic methods
}
OrderItem Entity:
- Attributes:
orderItemId
(Unique identifier)product
(Product included in the order)quantity
subtotal
- Characteristics:
- Immutable once created (orderItemId remains constant)
- Represents a specific product within an order
- Encapsulates business rules, such as calculating subtotal
- Relationships:
- Connected to a
Product
entity - Part of an
Order
entity
- Connected to a
public class OrderItem {
private final UUID orderItemId;
private final Product product;
private final int quantity;
private final BigDecimal subtotal;
// Constructor, getters, and business logic methods
}
In this example, each entity encapsulates its own unique identity and encapsulates the related business logic. The immutability of certain attributes, such as productId
and orderId
, ensures consistency and clarity within the domain model. These entities, when combined, form a cohesive representation of the e-commerce domain in line with Domain-Driven Design principles.
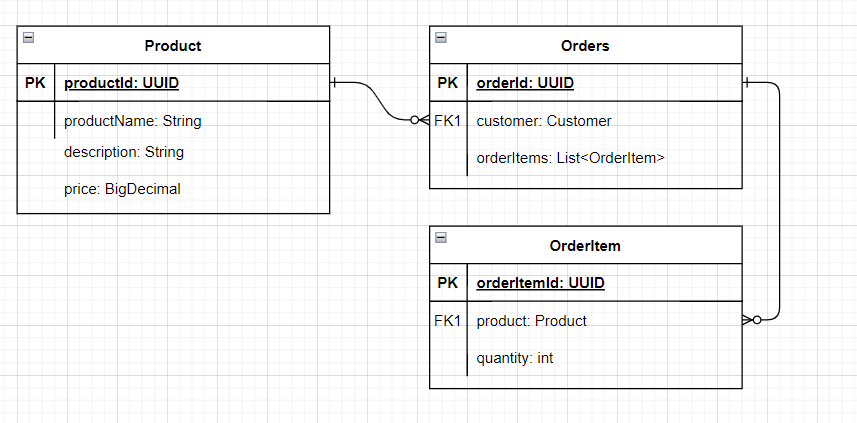
Benefits of Domain-Driven Design Entities
The use of DDD entities brings several advantages to software development. By encapsulating business logic within entities, code becomes more readable and maintainable. The unique identity of entities facilitates tracking changes and ensures a consistent representation of the domain model across the entire application.
Common Mistakes in Defining Domain-Driven Design Entities
While working with DDD entities, it’s essential to be aware of common pitfalls. Overcomplicating entity structures, neglecting immutability, or failing to establish clear relationships can lead to challenges down the line. To avoid these issues, developers should adhere to best practices and continuously refine their understanding of the domain.
Implementing Domain-Driven Design Entities in Real Projects
Real-world applications provide valuable insights into the practical implementation of DDD entities. Case studies of successful projects highlight the benefits and challenges faced during the development process. Learning from these experiences contributes to a more informed and effective use of DDD entities in new projects.
Tools and Frameworks for Domain-Driven Design Entities
Several tools and frameworks support the implementation of DDD principles, making it easier for developers to work with entities. These tools often provide abstractions that simplify entity management, allowing developers to focus on the core business logic. Familiarizing yourself with these tools can significantly enhance your DDD workflow.
- Hibernate:
- Description: Hibernate is a widely-used Java-based framework for object-relational mapping (ORM). It simplifies database interactions and supports the creation and management of DDD entities.
- Key Features:
- Automatic generation of SQL queries.
- Support for transparent persistence of objects.
- Entity Framework (EF):
- Description: Entity Framework is an ORM framework developed by Microsoft for .NET applications. It enables developers to work with DDD entities in a seamless manner, abstracting the underlying database operations.
- Key Features:
- Code-first and database-first approaches.
- Support for LINQ queries.
- Spring Data JPA:
- Description: Spring Data JPA is part of the larger Spring Data project and simplifies data access in Java applications. It integrates with the Java Persistence API (JPA) to handle DDD entities.
- Key Features:
- Automatic query generation.
- Repository support for entity management.
- Axon Framework:
- Description: Axon Framework is a Java-based framework specifically designed for building scalable and distributed applications using DDD principles. It provides infrastructure support for handling commands, events, and aggregates.
- Key Features:
- CQRS (Command Query Responsibility Segregation) support.
- Event Sourcing capabilities.
- DDDLite:
- Description: DDDLite is a lightweight framework for Domain-Driven Design in Java. It focuses on simplicity and ease of use, providing a set of annotations and conventions for DDD entities.
- Key Features:
- Simple and intuitive API.
- Annotations for aggregate roots, entities, and value objects.
- Laravel Eloquent (for PHP):
- Description: Laravel Eloquent is an ORM included with the Laravel PHP framework. It simplifies database interactions and supports the definition and usage of DDD entities.
- Key Features:
- Fluent query builder.
- Eloquent relationships for entity associations.
- DDD4J:
- Description: DDD4J is a Domain-Driven Design framework for Java. It provides abstractions and base classes to help developers implement DDD concepts such as aggregates and repositories.
- Key Features:
- Base classes for entities, value objects, and aggregates.
- Repositories with common DDD patterns.
- Microsoft.EntityFrameworkCore (for .NET Core):
- Description: Microsoft.EntityFrameworkCore is the Entity Framework Core library for .NET Core applications. It extends Entity Framework to support cross-platform development and works seamlessly with DDD entities.
- Key Features:
- Cross-platform compatibility.
- Asynchronous query execution.
Future Trends in Domain-Driven Design Entities
As technology continues to evolve, the role of DDD entities is likely to undergo changes. Emerging trends, such as microservices architecture and serverless computing, impact how entities are designed and managed. Staying abreast of these trends is essential for developers looking to future-proof their applications.
Conclusion
In conclusion, DDD entities form the backbone of domain-driven software development. Understanding their significance, features, and best practices for implementation is crucial for building successful applications. As technology advances, the role of entities will continue to evolve, and developers must adapt to these changes to stay at the forefront of the industry.
Frequently Asked Questions (FAQs)
- What is the primary purpose of DDD entities in software development?
- DDD entities play a crucial role in representing and encapsulating core business concepts in a way that enhances code readability and maintainability.
- How can developers avoid common mistakes when defining DDD entities?
- By adhering to best practices, such as keeping entities immutable, clearly defining relationships, and continuously refining the understanding of the domain.
- Are there specific tools recommended for working with DDD entities?
- Several tools and frameworks support DDD principles, including Hibernate, Entity Framework, and Axon Framework. The choice depends on the technology stack and project requirements.
- Can DDD entities be used in conjunction with microservices architecture?
- Yes, DDD entities are compatible with microservices architecture and can be a valuable component in designing scalable and maintainable distributed systems.
- How do DDD entities contribute to code maintainability?
- By encapsulating business logic within entities, changes to the domain can be localized, making the codebase more modular and easier to maintain.
Thank you for reading! If you have any more questions or need further clarification, feel free to reach out.