Best Way to Optimizing Stored Procedures in SQL Server : Basic
Contents
Article: Optimizing Stored Procedures in SQL Server
In the dynamic world of database management, optimizing stored procedures in SQL server is a critical aspect of ensuring optimal performance for applications relying on SQL Server. Let’s delve into the intricacies of this process, understanding its significance and exploring effective strategies.
Introduction of Optimizing Stored Procedures in SQL Server
Database management, the efficiency of stored procedures plays a pivotal role in determining the overall performance of an application. SQL Server, a robust and widely used relational database management system, demands careful attention to the optimization of stored procedures to ensure seamless operation and enhanced user experience.
Understanding Stored Procedures
Definition and Purpose
Stored procedures are precompiled sets of one or more SQL statements that are stored for reuse. They offer a way to modularize database logic, promoting code reusability and maintainability. However, without proper optimization, they can become bottlenecks in the system.
Common Challenges in Optimization
As applications grow in complexity, stored procedures face challenges such as increased execution time and resource consumption. These challenges highlight the need for a thoughtful optimization strategy.
Benefits of Optimization
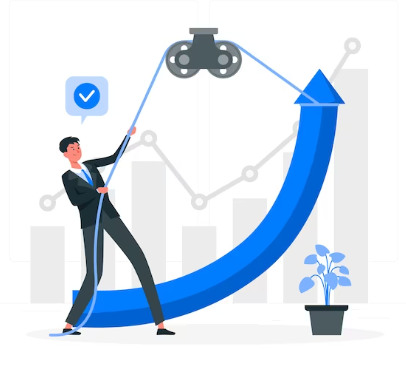
Improved Query Performance
One of the primary advantages of optimizing stored procedures is the significant improvement in query performance. By fine-tuning the logic and structure of these procedures, developers can reduce execution times and enhance overall responsiveness.
Use Indexes:
- Create indexes on columns used in WHERE clauses and JOIN conditions.
CREATE INDEX idx_employee_name ON employee(name);
Limit the Number of Rows Fetched:
- Use the
LIMIT
clause to restrict the number of rows returned, especially when you don’t need the entire result set.
SELECT * FROM orders LIMIT 10;
*Avoid SELECT :
- Instead of selecting all columns, only retrieve the columns you need. This reduces data transfer and improves performance.
SELECT order_id, customer_name FROM orders;
Use EXISTS and IN efficiently:
- Use
EXISTS
andIN
clauses judiciously, as they can be resource-intensive.
SELECT * FROM products WHERE category_id IN (SELECT category_id FROM categories WHERE category_name = 'Electronics');
Optimize JOINs:
- Use the appropriate JOIN types (INNER, LEFT, RIGHT) based on your needs.
SELECT customers.customer_id, customers.name, orders.order_id
FROM customers
INNER JOIN orders ON customers.customer_id = orders.customer_id;
Avoid Using Functions in WHERE Clause:
- Applying functions to columns in the WHERE clause can prevent index usage.
-- Less efficient
SELECT * FROM products WHERE YEAR(order_date) = 2022;
-- More efficient
SELECT * FROM products WHERE order_date >= '2022-01-01' AND order_date < '2023-01-01';
Use Proper Data Types:
- Choose appropriate data types for columns to save storage and improve performance.
CREATE TABLE employees (
employee_id INT,
name VARCHAR(255),
hire_date DATE
);
Enhanced Database Scalability
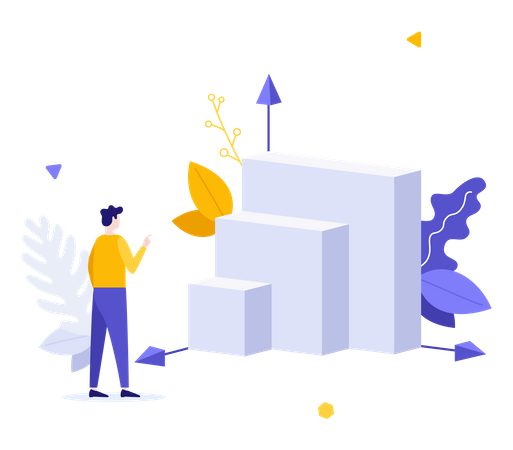
Optimized stored procedures contribute to better scalability, allowing applications to handle a growing number of users and increasing data volumes. This scalability is crucial for applications experiencing expansion or sudden surges in usage.
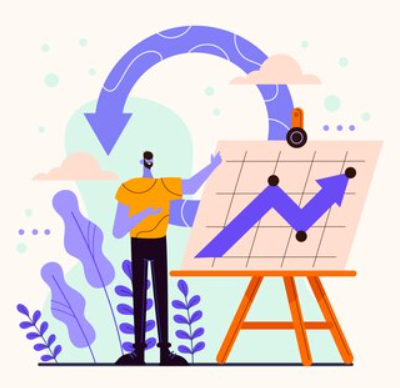
Better Resource Utilization
Optimization leads to more efficient use of system resources, preventing unnecessary strain on the server. This, in turn, translates to cost savings and a smoother user experience.
Identifying Performance Bottlenecks
Profiling Tools for SQL Server
Profiling tools like SQL Server Profiler provide insights into the performance of stored procedures by capturing and analyzing events during their execution. This helps developers pinpoint areas that require optimization.
Analyzing Execution Plans
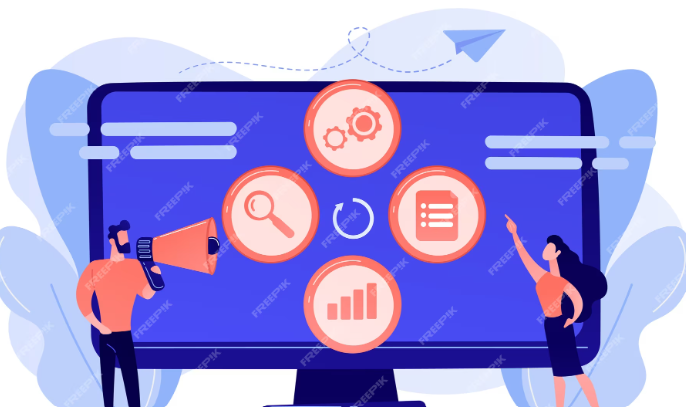
Examining execution plans through tools like SQL Server Management Studio (SSMS) allows a detailed view of how stored procedures are processed. Identifying inefficient query plans is crucial for targeted optimization.
Here is an example of how you can retrieve actual data from the execution plan in SQL Server:
-- Enable the XML execution plan output
SET STATISTICS XML ON;
-- Your SQL query goes here
SELECT * FROM YourTableName WHERE YourCondition;
-- Disable the XML execution plan output
SET STATISTICS XML OFF;
When you run this query, SQL Server will provide the execution plan in XML format along with the actual data. You can then review the execution plan to identify areas for optimization.
Alternatively, you can use tools like SQL Server Management Studio (SSMS) to view graphical execution plans, making it easier to analyze and optimize queries visually. To view the execution plan in SSMS:
- Open SSMS and connect to your database.
- Open a new query window.
- Type or paste your SQL query in the window.
- Click on the “Include Actual Execution Plan” button (or press Ctrl + M) before executing the query.
- Execute the query.
The graphical execution plan will be displayed in a separate tab, allowing you to analyze the flow of the query and identify potential performance bottlenecks.
Keep in mind that optimizing queries involves various factors, such as index usage, statistics, and query structure. The execution plan, whether in XML or graphical form, is a valuable tool for understanding how the database engine processes your queries and making informed decisions to improve performance.
Monitoring Resource Usage
Regularly monitoring resource usage, including CPU, memory, and disk I/O, is essential for understanding the impact of stored procedures on the overall system. Tools like Resource Governor aid in maintaining resource allocation balance.
Techniques for Optimizing Stored Procedures
Indexing Strategies
Strategic indexing is a cornerstone of stored procedure optimization. Properly indexed tables significantly reduce query execution times by facilitating quicker data retrieval.
- Single-Column Index:
- Create an index on a single column.
CREATE INDEX idx_name ON users (name);
- Composite Index:
- Create an index on multiple columns.
CREATE INDEX idx_name_age ON users (name, age);
- Unique Index:
- Ensure uniqueness using a unique index.
CREATE UNIQUE INDEX idx_email ON employees (email);
- Clustered Index:
- Organize the data on the disk based on the index.
CREATE CLUSTERED INDEX idx_date ON orders (order_date);
- Covering Index:
- Include all columns needed for a query in the index.
CREATE INDEX idx_covering ON products (category, price) INCLUDE (name, stock);
- Partial Index:
- Index a subset of the data based on a condition.
CREATE INDEX idx_active_users ON accounts (user_id) WHERE is_active = true;
- Function-Based Index:
- Index based on a function or expression.
CREATE INDEX idx_name_length ON customers (LENGTH(name));
- Foreign Key Index:
- Index foreign keys for join optimization.
CREATE INDEX idx_fk_user_id ON orders (user_id);
- Bitmap Index:
- Suitable for low cardinality columns.
CREATE BITMAP INDEX idx_status ON tasks (status);
- Spatial Index:
- For spatial data types (e.g., geometry, geography).
CREATE SPATIAL INDEX idx_location ON locations (coordinate);
Query Rewriting and Restructuring
Optimizing the logic within stored procedures involves scrutinizing and rewriting queries for efficiency. Restructuring queries can lead to improved execution plans and better overall performance.
Parameter Optimization
Carefully tuning parameters within stored procedures ensures that queries are optimized for specific use cases. This involves considering the data distribution and cardinality of parameters.
Caching Mechanisms
Implementing caching mechanisms, such as memoization, can drastically reduce the need for repetitive and resource-intensive calculations within stored procedures.
Best Practices
Regular Performance Monitoring
Frequent monitoring of stored procedure performance is crucial for identifying issues before they escalate. Establishing a routine for performance checks helps maintain an optimized database environment.
Utilizing Stored Procedure Templates
Developing and adhering to standardized stored procedure templates ensures consistency across the database. This simplifies optimization efforts and aids in maintaining a uniform coding structure.
Version Control and Documentation
Implementing version control and comprehensive documentation practices ensures that changes to stored procedures are tracked and understood. This transparency is vital for collaborative development and troubleshooting.
Case Studies
Real-World Examples of Successful Optimization
Examining real-world case studies provides valuable insights into the tangible benefits of stored procedure optimization. Success stories showcase the transformative impact on application performance.
Impact on Application Performance
Illustrating the direct correlation between optimized stored procedures and enhanced application performance emphasizes the practical advantages for developers and end-users alike.
Common Mistakes to Avoid
Overlooking Indexing
Neglecting the importance of proper indexing can lead to sluggish query performance. Developers must prioritize indexing strategies to unlock the full potential of stored procedure optimization.
Ignoring Parameterization
Failing to optimize and parameterize queries within stored procedures can result in suboptimal execution plans. Parameterization allows for better plan reuse and adaptable query optimization.
Lack of Regular Optimization Efforts
Treating optimization as a one-time task rather than an ongoing process can hinder long-term database health. Regular optimization efforts are essential for adapting to changing usage patterns and data volumes.
Future Trends in Stored Procedure Optimization
Machine Learning Applications
The integration of machine learning algorithms in stored procedure optimization is an emerging trend. These applications can learn from historical performance data to suggest and implement optimization strategies.
Automation in Optimization Processes
The future holds increased automation in the optimization of stored procedures. Automated tools and scripts will streamline the optimization process, reducing the manual effort required.
Challenges and Solutions
Dealing with Legacy Systems
Adapting optimization strategies to legacy systems poses challenges due to outdated technologies and architecture. However, incremental improvements and careful planning can overcome these obstacles.
Balancing Optimization and Development Speed
Striking a balance between optimizing stored procedures in SQL server and maintaining development speed is crucial. Developers must find efficient ways to incorporate optimization without compromising agility.