T-SQL Data Types: A Comprehensive Guide
In SQL Server, every column, native variable, expression, and parameter has their own data type. T-SQL Data Type is an attribute that specifies the type of data that the object can hold: character data, floating data integer data, monetary data, date and time data, binary strings, and so on.
Contents
Exploring T-SQL Data Types for Enhanced Database Management
In the realm of database design, the significance of choosing the right data types cannot be overstated. Let’s embark on a journey through the T-SQL data types landscape, unraveling the potential they hold for database administrators and developers alike.
The Foundation: Basic T-SQL Data Types
To build a robust database foundation, one must first grasp the basics. T-SQL offers a range of fundamental data types, each serving a unique purpose. From integers to decimals, understanding these foundational elements is key to crafting a well-structured database schema.
- Integers (int, smallint, bigint):
- Example:
int
can store whole numbers like 123, -456, or 7890. It is commonly used for storing numerical data without decimals. - Usage: Ideal for representing counts, identifiers, or any scenario where decimal precision is not required.
- Example:
- Decimals (numeric, decimal):
- Example:
decimal(8, 2)
can store values like 12345.67, providing precision up to two decimal places. - Usage: Suitable for financial data or any situation where accurate decimal representation is essential.
- Example:
- Floating-Point Numbers (float, real):
- Example:
float
can store numbers like 123.456789, accommodating a wide range of values. - Usage: Useful for scientific calculations or scenarios where a broader range of numerical values is expected.
- Example:
- Date and Time (date, time, datetime):
- Example:
datetime
can represent a specific date and time, such as ‘2024-03-10 15:30:00’. - Usage: Essential for applications requiring temporal data, like transaction timestamps or scheduling events.
- Example:
- Boolean (bit):
- Example:
bit
can store either 0 or 1, representing true or false. - Usage: Ideal for binary choices, such as indicating the status of a process (e.g., active/inactive).
- Example:
- Character Strings (char, varchar, nchar, nvarchar):
- Example:
varchar(50)
can store variable-length character strings like ‘Hello, World!’. - Usage: Commonly used for storing textual information, such as names, addresses, or descriptions.
- Example:
- Binary (binary, varbinary):
- Example:
varbinary(max)
can store binary data like images or documents. - Usage: Suitable for scenarios involving the storage of raw binary information.
- Example:
Understanding these basic T-SQL data types is crucial for designing a database schema that accurately represents and efficiently handles your data. Whether you’re working with integers, decimals, dates, or strings, choosing the right data type ensures optimal storage and retrieval, contributing to the overall performance and reliability of your database system.
T-SQL Data Type example
Lets consider example how to declare variable and table column
Declare variable and Table Column with Data Type
declare @variableName DataType declare @varName varchar(500) CREATE TABLE Table1 ( Column1 int )
Exact Numeric Types
Data Type | From | To |
---|---|---|
bigint | -9,223,372,036,854,775,808 | 9,223,372,036,854,775,807 |
int | -2,147,483,648 | 2,147,483,647 |
smallint | -32,768 | 32,767 |
tinyint | 0 | 255 |
bit | 0 | 1 |
decimal | -10^38 +1 | 10^38 –1 |
numeric | -10^38 +1 | 10^38 –1 |
money | -922,337,203,685,477.5808 | +922,337,203,685,477.5807 |
smallmoney | -214,748.3648 | +214,748.3647 |
Approximate numerics
Data Type | From | To |
---|---|---|
Float | -1.79E + 308 | 1.79E + 308 |
Real | -3.40E + 38 | 3.40E + 38 |
Date And Time
Date Type | From | To |
---|---|---|
datetime(3.33 milliseconds accuracy) | Jan 1, 1753 | Dec 31, 9999 |
smalldatetime(1 minute accuracy) | Jan 1, 1900 | Jun 6, 2079 |
date(1 day accuracy. Introduced in SQL Server 2008) | Jan 1, 0001 | Dec 31, 9999 |
datetimeoffset(100 nanoseconds accuracy. Introduced in SQL Server 2008) | Jan 1, 0001 | Dec 31, 9999 |
datetime2(100 nanoseconds accuracy. Introduced in SQL Server 2008) | Jan 1, 0001 | Dec 31, 9999 |
time(100 nanoseconds accuracy. Introduced in SQL Server 2008) | 00:00:00.0000000 | 23:59:59.9999999 |
Unicode Character Strings
Data Type | Description |
---|---|
nchar | Fixed-length Unicode data. Maximum length of 4,000 characters. |
nvarchar | Variable-length Unicode data. Maximum length of 4,000 characters. |
Nvarchar (max) | Variable-length Unicode data. Maximum length of 230 characters (Introduced in SQL Server 2005). |
ntext | Variable-length Unicode data. Maximum length of 1,073,741,823 characters. |
Binary Strings
Data Type | Description |
---|---|
binary | Fixed-length binary data. Maximum length of 8,000 bytes. |
varbinary | Variable-length binary data. Maximum length of 8,000 bytes. |
varbinary(max) | Variable-length binary data. Maximum length of 231 bytes (Introduced in SQL Server 2005). |
image | Variable-length binary data. Mmaximum length of 2,147,483,647 bytes. |
Other Data Types
Data Type | Description |
---|---|
sql_variant | Stores values of various SQL Server-supported data types, except text, ntext, and timestamp. |
timestamp | Stores a database-wide unique number that gets updated every time a row gets updated. |
uniqueidentifier | Stores a globally unique identifier (GUID). |
xml | Stores XML data. Store XML instances in a column or a variable (Introduced in SQL Server 2005). |
cursor | A reference to a cursor. |
table | Stores a result set for later processing. |
hierarchyid | A variable length, system data type used to represent position in a hierarchy (Introduced in SQL Server 2008). |
Optimizing Storage with Numeric and Decimal Data Types
In the quest for efficient storage, leveraging numeric and decimal data types becomes crucial. Discover how these data types contribute to precision in calculations while minimizing storage overhead. Unearth the secrets to optimizing your database storage and computation power.
Navigating Character Data: Strings in T-SQL
Strings play a pivotal role in database management, and T-SQL provides a versatile array of character data types. Explore the intricacies of working with char, varchar, and text, unraveling the potential for efficient storage and retrieval of textual information.
CHAR and VARCHAR:
- CHAR: This fixed-length character data type is suitable for storing strings with a constant length. For example, if you have a column for storing country codes, where each code is always three characters long, CHAR could be used.
- VARCHAR: Unlike CHAR, VARCHAR is a variable-length character data type. It’s more flexible, as it only stores the actual data and doesn’t pad it with spaces. If your data has varying lengths, like storing names of different lengths, VARCHAR is a more efficient choice.
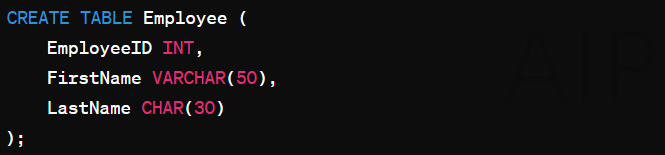
Temporal Data Types: Managing Time Effectively
Time management extends beyond personal productivity—it’s a critical aspect of database design. T-SQL equips developers with temporal data types, offering efficient ways to handle dates and times. Learn how to manage temporal data seamlessly, ensuring accuracy and precision in your database applications.
Beyond Basics: User-Defined Data Types in T-SQL
Elevate your database design to new heights by delving into the realm of user-defined data types. Understand how these customizable data types empower developers to encapsulate complex structures, promoting code reusability and enhancing overall system maintainability.
Enhancing Performance with Binary and Image Data Types
In the digital age, dealing with binary data is inevitable. T-SQL’s binary and image data types open doors to efficient storage and retrieval of binary information. Unlock the potential for enhancing performance in scenarios involving multimedia or large binary objects.
Efficient Querying with T-SQL Data Type Functions
Mastering T-SQL data type functions is a game-changer for optimizing your database queries. Dive into the world of conversion and manipulation functions, gaining the skills to transform and extract information seamlessly.
1. Conversion Functions:
Consider a scenario where you have a date stored in a string format, and you need to convert it to a datetime data type for better manipulation. The T-SQL CONVERT
function comes into play:
SELECT CONVERT(DATETIME, '2022-03-10', 120) AS ConvertedDate;
Here, the CONVERT
function transforms the string ‘2022-03-10’ into a datetime data type using the format code 120.
2. Manipulation Functions:
Suppose you want to concatenate two string columns in a table to create a full name. The CONCAT
function simplifies this operation:
SELECT CONCAT(FirstName, ' ', LastName) AS FullName
FROM Customers;
3. String Manipulation Functions:
Consider a scenario where you need to extract a specific portion of a string, such as extracting the domain from an email address. The SUBSTRING
and CHARINDEX
functions can help:
SELECT SUBSTRING(EmailAddress, CHARINDEX('@', EmailAddress) + 1, LEN(EmailAddress)) AS Domain
FROM Users;
In this example, SUBSTRING
extracts the domain portion of the ‘EmailAddress’ column by finding the position of ‘@’ using CHARINDEX
.
Conclusion: Harnessing the Power of T-SQL Data Types
In conclusion, the world of T-SQL data types is a realm of immense possibilities for developers and database administrators. By understanding and leveraging these data types effectively, you not only enhance your database performance but also elevate the overall efficiency of your applications. Stay ahead in the ever-evolving landscape of database management with the knowledge and insights gained from this comprehensive guide.