In this tutorial, we are using VS Code to write code
To write, compile, and run a Rust: Write HelloWorld program using Visual Studio Code (VS Code), follow these steps
Contents
Prerequisites
- Install Rust: Make sure Rust is installed on your system. You can verify this by running
rustc --version
andcargo --version
in your terminal. If not installed, follow the previous instructions to install Rust on your computer. - Install VS Code: Download and install Visual Studio Code from the official website.
Create a New Rust Project
- Open a terminal in VS Code by selecting
Terminal
>New Terminal
from the main menu or by pressingCtrl+
(Windows/Linux) orCmd+
(macOS). - Go to the directory where you want to create your new Rust project.
- Run the below command to create a new Rust project named
hello_world
cargo new hello_world
- This will create a new directory named
hello_world
with a basic Rust project structure.
Open the Project in VS Code
- In the terminal, navigate to the new project directory
cd hello_world
- Open the project in VS Code by running
code .
Write the “Hello, World!” Code:
- VS Code should automatically open the
src/main.rs
file. If not, navigate to thesrc
folder and openmain.rs
. - You should see the default “Hello, World!” program. It looks like this
fn main() {
println!("Hello world !!!");
}
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello world !!!");
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Hello world !!!");
}
}
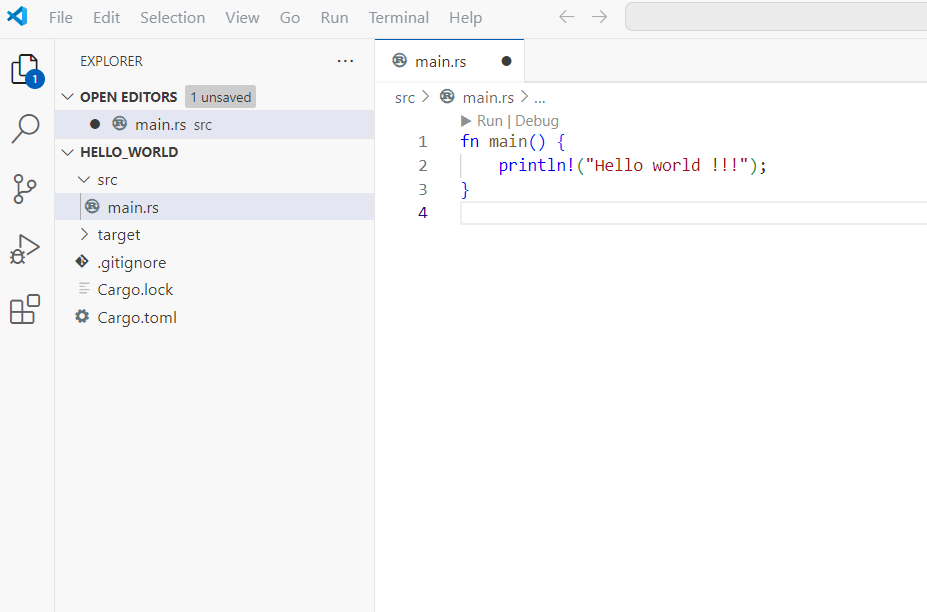
Build and Run the Program
- Open the terminal in VS Code.
- Make sure you are in the root directory of your Rust project (where
Cargo.toml
is located). - Run the following command to build and run your Rust program
cargo run
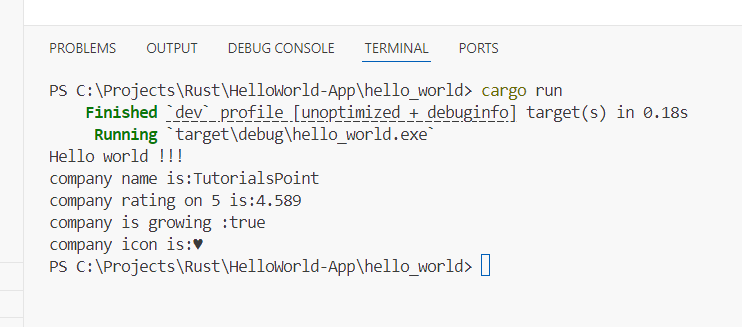