JavaScript Enter only numbers in the text box Validation: Free Guide
Contents
In the world of web development, ensuring data integrity and user experience are paramount. One common challenge developers face is restricting input fields to accept only numeric values. This ensures accurate data processing and prevents errors down the line. In this article, we’ll explore the importance of allowing Enter only numbers in the text box and effective methods to achieve this in your web applications.
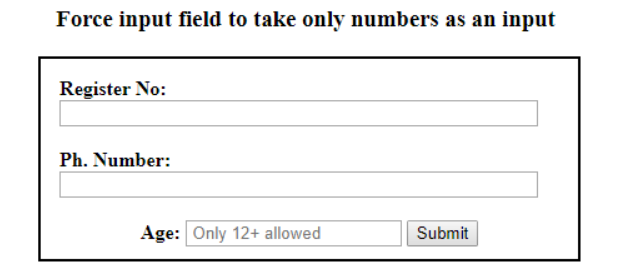
Methods for Implementing Numeric Input Validation
There are several approaches developers can take to implement numeric input validation in text boxes. Let’s explore some of the most effective methods:
1. HTML Input Attributes
HTML provides attributes such as type="number"
that can be applied to input fields to specify that only numeric values are allowed. This method leverages built-in browser functionality to validate input on the client-side, providing instant feedback to users.
2. JavaScript Validation
For more flexibility and customization, developers can use JavaScript to validate input dynamically. By attaching event listeners to input fields, developers can intercept user input and validate it against predefined criteria. This approach allows for real-time validation and custom error messaging tailored to the specific input field.
3. Regular Expressions (Regex)
Regular expressions offer a powerful way to define complex patterns for validating input. Developers can create regex patterns that match numeric values and use them to validate user input in text boxes. While regex provides extensive flexibility, it may require a deeper understanding and can be more complex to implement compared to other methods.
Example: Enter only numbers in the text box Validation
function EnterOnlyNumbers(e) {
var theEvent = e.htmlEvent || window.event;
var key = theEvent.keyCode || theEvent.which;
key = String.fromCharCode(key);
var regex = /[0-9,b]|./;
if (!regex.test(key)) {
theEvent.returnValue = false;
if (theEvent.preventDefault)
theEvent.preventDefault();
}
}
Best Practices for Numeric Input Validation
Regardless of the method chosen, there are some best practices developers should follow when implementing numeric input validation:
- Provide clear and concise error messages that inform users about the expected input format.
- Validate input on both the client and server-side to ensure robust data validation.
- Consider accessibility requirements and ensure that error messages are communicated effectively to all users, including those using assistive technologies.
- Test input validation thoroughly across different browsers and devices to ensure consistent behavior.
Conclusion
In conclusion, enter only numbers in the text box is essential for maintaining data integrity and enhancing the user experience in web applications. By implementing effective validation methods, developers can prevent errors, improve usability, and ultimately create a more reliable and user-friendly product. Whether leveraging HTML attributes, JavaScript validation, or regular expressions, prioritizing numeric input validation is a crucial step towards building high-quality web applications.