How Embed an image in a PDF file using C#: Easy Way
When we develop the system, sometime customer want to embed an image in a PDF file. image may be Stamp, Signature and etc.. Using Visual Studio C# we can easily meet the above requirement. Doing this requirement we want to X509Certificate2 file
Understanding the Importance of Image Embedding in PDFs
Image embedding in PDF files serves a multitude of purposes, from enhancing the visual appeal of documents to providing vital information through graphics and illustrations. Whether you’re creating reports, presentations, or educational materials, incorporating images can significantly elevate the quality and effectiveness of your content.
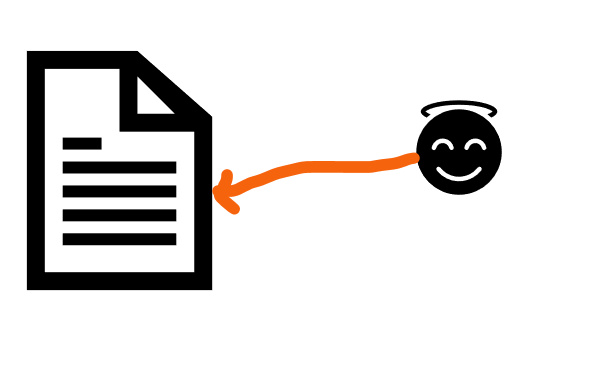
Getting Started with C# for PDF Manipulation
Before we delve into the intricacies of embedding images, it’s essential to have a basic understanding of C# programming and PDF manipulation. C# is a versatile and powerful programming language commonly used for developing applications, including those that involve PDF generation and manipulation.
Exploring the Libraries for PDF Manipulation in C#
To embed images in PDF files using C#, we’ll leverage libraries that offer comprehensive support for PDF manipulation. Some popular options include iTextSharp, PDFSharp, and Syncfusion Essential PDF. These libraries provide extensive functionality for creating, modifying, and managing PDF documents programmatically.
Contents
Step-by-Step Guide to Embed an Image in a PDF File
Now, let’s walk through the process of embedding images in PDF files using C#:
- Install the Necessary Libraries: Begin by installing the preferred PDF manipulation library via NuGet Package Manager in Visual Studio. This will allow you to easily incorporate the library into your C# project.
- Initialize the PDF Document: Create a new PDF document object using the chosen library. This serves as the foundation for adding content, including text, images, and other elements.
- Load the Image to Embed: Load the image file that you want to embed into the PDF document. Ensure that the image file is accessible and properly formatted to ensure seamless integration.
- Add the Image to the PDF Document: Utilize the appropriate method provided by the PDF manipulation library to add the loaded image to the PDF document. Specify the position, size, and other relevant properties of the image within the document.
- Save the Modified PDF Document: Once the image has been successfully embedded, save the modified PDF document to preserve the changes. Choose the desired file path and format for the output PDF file.
Function
String mUploadFilePath = “FIlePath/PDFFileName.pdf”;
documentProcessor.LoadDocument(mUploadFilePath);
X509Certificate2 certificate = new X509Certificate2(System.Web.HttpContext.Current.Server.MapPath(CertificateFileName), Password);
byte[] imageData = File.ReadAllBytes(System.Web.HttpContext.Current.Server.MapPath(“Embed Image File Path”));
int pageNumber = 1;
int angleInDegrees = 45;
double angleInRadians = angleInDegrees * (Math.PI / 180);
PdfOrientedRectangle signatureBounds = new PdfOrientedRectangle(new PdfPoint(305, 88), 250, 80, 0);
PdfSignature signature = new PdfSignature(certificate, imageData, pageNumber, signatureBounds);
using (SolidBrush textBrush = new SolidBrush(Color.FromArgb(100, Color.Blue)))
documentProcessor.SaveDocument(“Save New File Path/FileName.pdf”, new PdfSaveOptions() { Signature = signature });
Sample X509Certificate2 file
Above I attached sample Certificate file for you easy reference.
Optimizing Image Embedding for Performance and Quality
When embedding images in PDF files, it’s essential to consider factors such as file size, resolution, and compression to optimize performance and maintain quality. Experiment with different settings and techniques to strike the right balance between visual appeal and file size.
- File Size:
- Choose image formats wisely. Formats like JPEG are suitable for photographs with many colors, while PNG is ideal for images with transparency or sharp lines.
- Utilize compression techniques to reduce file size without compromising image quality. Most PDF manipulation libraries offer options for image compression.
- Resolution:
- Consider the intended use of the PDF document. For documents primarily viewed on screens, a resolution of 72 or 96 DPI (dots per inch) is sufficient. For printing purposes, aim for a higher resolution of 300 DPI or more.
- Resize images to fit their intended display size within the PDF document. Avoid embedding excessively large images that may inflate file size unnecessarily.
- Color Space:
- Convert images to the appropriate color space based on the document’s requirements. For full-color images, use RGB (Red, Green, Blue) color space. For documents with strict color accuracy requirements, such as print-ready materials, opt for CMYK (Cyan, Magenta, Yellow, Black) color space.
- Compression:
- Explore different compression algorithms and settings provided by your PDF manipulation library. Balance the level of compression to achieve a smaller file size while maintaining acceptable image quality.
- Utilize lossless compression for images that require high fidelity and detail preservation. For images where some loss of quality is acceptable, consider using lossy compression algorithms.
- Image Optimization Tools:
- Leverage image optimization tools and software to preprocess images before embedding them into PDF files. These tools can automatically apply compression, resize images, and convert to optimal formats, streamlining the optimization process.
- Testing and Iteration:
- Conduct thorough testing of the PDF document across different devices and platforms to ensure optimal performance and quality. Pay attention to factors such as loading times, visual clarity, and compatibility with various PDF viewers.
- Iterate on the optimization process based on feedback and performance metrics. Fine-tune compression settings, image formats, and resolution as needed to strike the perfect balance between performance and quality.
Conclusion
Incorporating images into PDF files using C# opens up a world of possibilities for creating dynamic and engaging documents. By following the steps outlined in this guide and leveraging the capabilities of PDF manipulation libraries, you can seamlessly integrate images into your PDFs and enhance the overall quality and effectiveness of your content. Unlock the full potential of your PDF documents with image embedding in C# today!