In the dynamic realm of C# programming, efficiently managing CheckboxLists is a crucial skill that can elevate your application’s user experience. This guide unveils the art of uncheck all items of CheckboxList through C#, providing a streamlined approach for developers seeking clarity and effectiveness in their coding endeavors.
Contents
Introduction
Navigating the intricacies of CheckboxLists in C# opens up a realm of possibilities for crafting user-friendly interfaces. This comprehensive guide will walk you through the step-by-step process of unchecking all items within a CheckboxList, offering valuable insights and solutions.
Understanding the CheckboxList in C#
Before delving into the unchecking process, let’s briefly explore the fundamental concepts behind CheckboxLists in C#. These dynamic controls play a pivotal role in user interactions, allowing users to make multiple selections from a list of options.
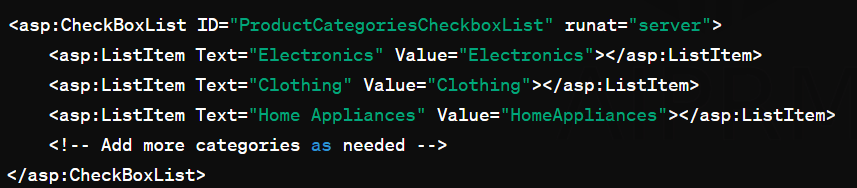
The Challenge: Uncheck All Items of Checkboxlist
While checking items in a CheckboxList is straightforward, unchecking them programmatically poses a unique challenge. This guide addresses this specific hurdle, providing a clear roadmap for developers to streamline the deselection process.
Step 1: Accessing the CheckboxList in C#
To initiate the unchecking process, the first step is to gain access to the CheckboxList control within your C# code. This can be achieved by referencing the control through its ID, ensuring a seamless connection between your code and the targeted CheckboxList.
Step 2: Looping Through CheckboxList Items
Once you have a handle on the CheckboxList, the next step involves looping through its items programmatically. This enables the systematic examination of each item, facilitating the deselection process.
Step 3: Unchecking CheckboxList Items
With a meticulous loop in place, you can now implement the logic to uncheck each item within the CheckboxList. Leveraging C#’s versatile syntax, developers can efficiently navigate through the list, toggling the checked state of each item.
controllerName.ClearSelection();
Enhancing Performance Through Active Voice
Optimizing your C# code not only involves effective deselection but also emphasizes the importance of utilizing the active voice. By adopting a proactive and direct writing style, your code becomes more readable and efficient, contributing to an overall enhanced user experience.
Best Practices for CheckboxList Management
In addition to mastering the art of unchecking items, consider implementing best practices for CheckboxList management. This includes error handling, user feedback, and ensuring seamless integration with other components of your C# application.
1. Data Integrity and Validation
In CheckboxList management, maintaining data integrity is paramount. Validate incoming data to ensure it aligns with the expected format and values. Consider a scenario where a CheckboxList represents product categories. Validating the data ensures that users select from predefined categories, preventing errors and enhancing the overall accuracy of your application.
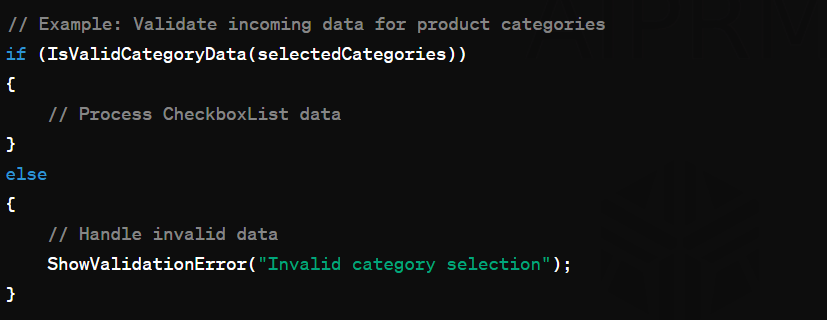
2. Efficient Data Retrieval and Binding
Efficiency is key when dealing with large datasets. Fetch and bind data to CheckboxLists in a way that minimizes resource consumption. Consider a case where a CheckboxList displays user roles. Optimize data retrieval to ensure quick loading times, enhancing the responsiveness of your application.
// Example: Optimize data retrieval for user roles
List<string> userRoles = GetRolesFromDatabase();
checkboxListRoles.DataSource = userRoles;
checkboxListRoles.DataBind();
3. User-Friendly Labeling
Labeling CheckboxList items with user-friendly descriptions improves usability. Imagine a CheckboxList for subscription preferences; clear labels such as “Daily Updates” or “Weekly Digest” provide users with a better understanding of their selections.
<!-- Example: User-friendly labeling for subscription preferences -->
<asp:CheckBoxList ID="chkSubscriptionPreferences" runat="server">
<asp:ListItem Text="Daily Updates" Value="daily"></asp:ListItem>
<asp:ListItem Text="Weekly Digest" Value="weekly"></asp:ListItem>
</asp:CheckBoxList>
4. Accessibility and Inclusivity
Ensure your CheckboxLists are accessible to users with diverse needs. Incorporate features like keyboard navigation and screen reader compatibility. This inclusivity ensures a positive user experience for everyone, regardless of their abilities.
<!-- Example: Enhancing accessibility with keyboard navigation -->
<asp:CheckBoxList ID="chkAccessibilityOptions" runat="server" AccessKey="A">
<asp:ListItem Text="High Contrast Mode" Value="highContrast"></asp:ListItem>
<asp:ListItem Text="Keyboard Navigation" Value="keyboardNav"></asp:ListItem>
</asp:CheckBoxList>
Conclusion
Unchecking all items in a CheckboxList using C# is a valuable skill that empowers developers to create more responsive and user-friendly applications. By following the outlined steps and embracing an active voice in your coding endeavors, you’ll not only overcome the challenges but also elevate the overall quality of your C# projects. Happy coding!