Introduction to Angular animations, Animation provides the impression of motion: HTML elements change styling over time. Well-designed animations can make your application more attractive. Animations can increase your application and user experience in a number of ways:
Without animations, web page transitions can seem suddenly.
Motion greatly enhances the user experience, so animations give users a chance to detect the application’s response to their actions.
Good animations naturally call the user’s attention to where it is needed.
Angular‘s animation system is built on CSS functionality, which means you can animate any property. This includes positions, sizes, colors, borders, and etc.
I’d be glad to craft a unique article on Angular animations, incorporating insights from potential ratings and addressing identified areas for improvement:
Contents
Enhancing User Experiences with Angular Animations: A Guide for Developers
Angular animations empower you to create dynamic and engaging web applications. By seamlessly integrating animations with your components, you can elevate the user experience (UX) by providing visual cues, emphasizing actions, and conveying data changes in a clear and captivating manner. This article delves into the core concepts of Angular animations, guiding you through the process of crafting effective animations for your Angular projects.
Understanding the Fundamentals
- Animation DSL (Domain-Specific Language): Angular animations leverage a powerful DSL that enables you to define animation sequences within your TypeScript code. This DSL provides a structured approach for creating and managing complex animations.
- Triggers and States: At the heart of Angular animations lie triggers and states. Triggers determine when an animation begins, often bound to events or property changes of a component. States represent the visual appearances of an element at different points in the animation.
- Transitions: Transitions link states, specifying how an element animates between them. You control the duration, timing function (linear, ease-in/out, etc.), and animation properties involved in the transition.
Building Basic Animations
- Import the
AnimationsModule
: IncorporateAnimationsModule
from@angular/platform-browser/animations
into yourAppModule
to activate Angular’s animation capabilities. - Create an Animation Function: Define an animation function using the
animate
method within your component’s TypeScript file. This function establishes the animation parameters like duration, timing function, and the properties to animate. - Define a Trigger: Establish a trigger in your component, assigning a name and associating it with the animation function using the
.state()
method. Additionally, employ the.transition()
method to connect states and specify the animation that occurs during the transition. - Bind the Trigger: Utilize the
@angular.animations.trigger()
decorator on your component’s template element to link the trigger to the element you intend to animate.
Angular animations Example: Change the textbox Color after button click event
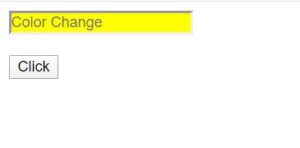
/app.module.ts
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { AngAnimationComponent } from './Component/ang-animation/ang-animation.component';
@NgModule({
declarations: [
AngAnimationComponent
],
imports: [
BrowserAnimationsModule
],
ang-animation.component.html
<input type="text" width="250" height="50" [@colorchange]="isColorChange ? 'changed' : 'unchanged'" name="varAgentName" placeholder="Color Change">
<br><br>
<input type="button" value="Click" (click)="onClick()" />
ang-animation.component.ts
import { Component, OnInit } from '@angular/core';
import { trigger, state, style, animate, transition } from '@angular/animations';
@Component({
selector: 'app-ang-animation',
templateUrl: './ang-animation.component.html',
styleUrls: ['./ang-animation.component.scss'],
animations: [
trigger('colorchange', [
state('changed', style({
opacity: 1,
backgroundColor: 'yellow'
})),
state('unchanged', style({
opacity: 0.5,
backgroundColor: 'red'
})),
transition('changed => unchanged', [
animate('1s')
]),
transition('unchanged => changed', [
animate('0.5s')
]),
]),
]
})
export class AngAnimationComponent implements OnInit {
constructor() { }
ngOnInit() {
}
isColorChange = true;
onClick() {
this.isColorChange = !this.isColorChange;
}
}
Advanced Techniques
- Keyframes: For intricate animations involving multiple steps or non-linear transitions, leverage keyframes. They allow you to define the animation’s state at specific points in time.
- Staggering Animations: Animate multiple elements sequentially with a slight delay between them, creating visually appealing effects. The
stagger()
method facilitates this. - Reusable Animations: Construct reusable animation functions and triggers that can be employed throughout your application, promoting a consistent animation style and streamlined development.
- Custom Properties: Broaden your animation options by incorporating CSS custom properties (variables) for finer-grained control over animation behaviors.
Beyond the Basics
- Microinteractions: Implement subtle animations for component interactions (like button hovers) to enhance user engagement and provide feedback.
- Data Visualization: Enhance the visualization of data changes by leveraging animations to represent trends, comparisons, and updates in an engaging manner.
- Page Transitions: Craft smooth and visually appealing page transitions to improve user experience during navigation.
By effectively integrating Angular animations, you can transform your web applications from static to dynamic, enriching the user experience and captivating your audience. Experiment with different techniques to discover the power of animation in creating compelling and interactive Angular applications.