Rust has become popular in programming because it’s all about keeping your work safe, running smoothly, and handling multiple tasks simultaneously. One of the coolest things about Rust is how it handles different types of collections. Whether you’re keeping track of a list of things, matching names with important info, or dealing with text, Rust has reliable and safe ways to manage your data. In this article, we’ll look at the most commonly used collections in Rust, like arrays, vectors, strings, string slices, and HashMaps.
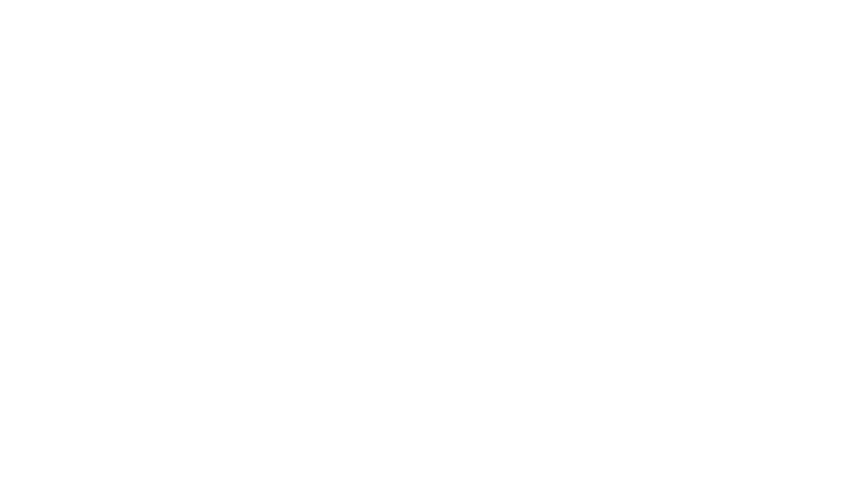
Contents
Understanding Collections in Rust
In Rust, collections are data structures that hold multiple values. They are essential for storing and managing data efficiently. Rust offers various types of collections, each with its unique characteristics and use cases. Let’s study these collections in detail.
Arrays in Rust
What Are Arrays?
Arrays in Rust are fixed-size sequences of elements of the same type. They are useful when you know the number of elements in advance and that number won’t change.
Declaring Arrays
Declaring an array in Rust is straightforward:
let numbers: [i32; 5] = [1, 2, 3, 4, 5];
Here, numbers is an array of five i32 integers.
Accessing Array Elements
You can access array elements using their index:
let first_number = numbers[0];
This code accesses the first element of the numbers array.
Array Length and Iteration
To get the length of an array, use the .len()
method:
let length = numbers.len();
To iterate over an array:
for number in &numbers {
println!("{}", number);
}
This loop prints each element in the array.

Vectors in Rust
What Are Vectors?
Vectors are similar to arrays but with a dynamic size. They are part of Rust’s standard library and allow you to grow or shrink the collection as needed.
Creating and Initializing Vectors
You can create a vector using the Vec
type:
let mut numbers: Vec<i32> = Vec::new();
numbers.push(1);
numbers.push(2);
Alternatively, you can use the vec! macro:
let numbers = vec![1, 2, 3, 4, 5];
Adding and Removing Elements
To add elements to a vector:
numbers.push(6);
To remove elements:
numbers.pop();
The pop
method removes the last element from the vector.
Iterating Over Vectors
Iterating over a vector is similar to iterating over an array:
for number in &numbers {
println!("{}", number);
}
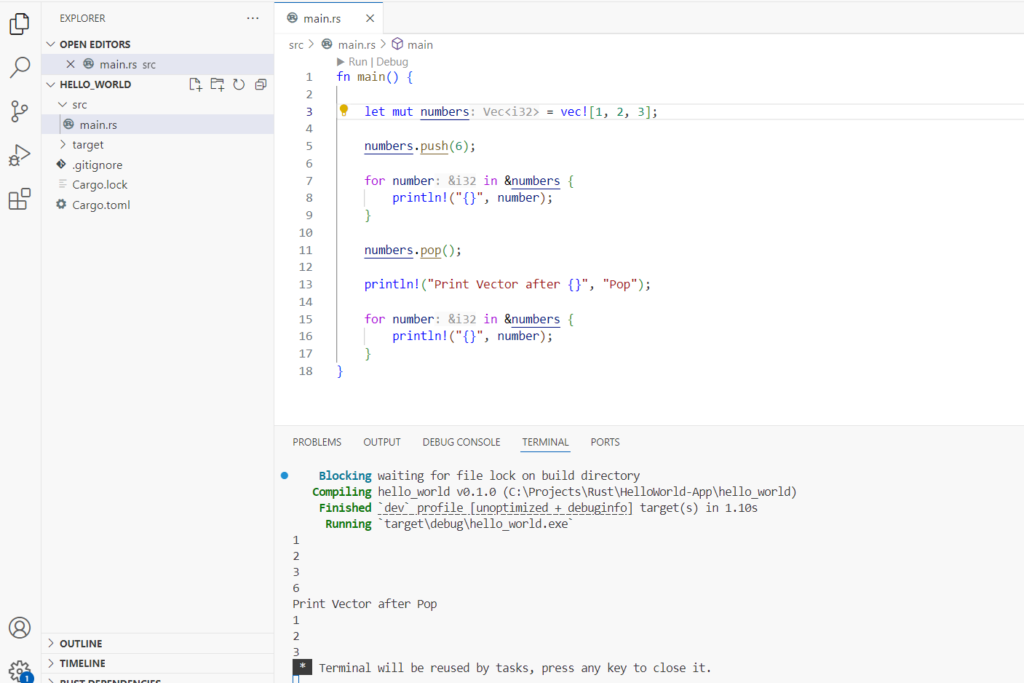
Strings and String Slices in Rust
Understanding Strings
What is a String?
In Rust, a String
is a growable, heap-allocated data structure used to store text.
Creating and Modifying Strings
Creating a String:
let mut s = String::from("Hello");
Modifying a String:
s.push_str(", world!");
This code appends ", world!" to the string s.
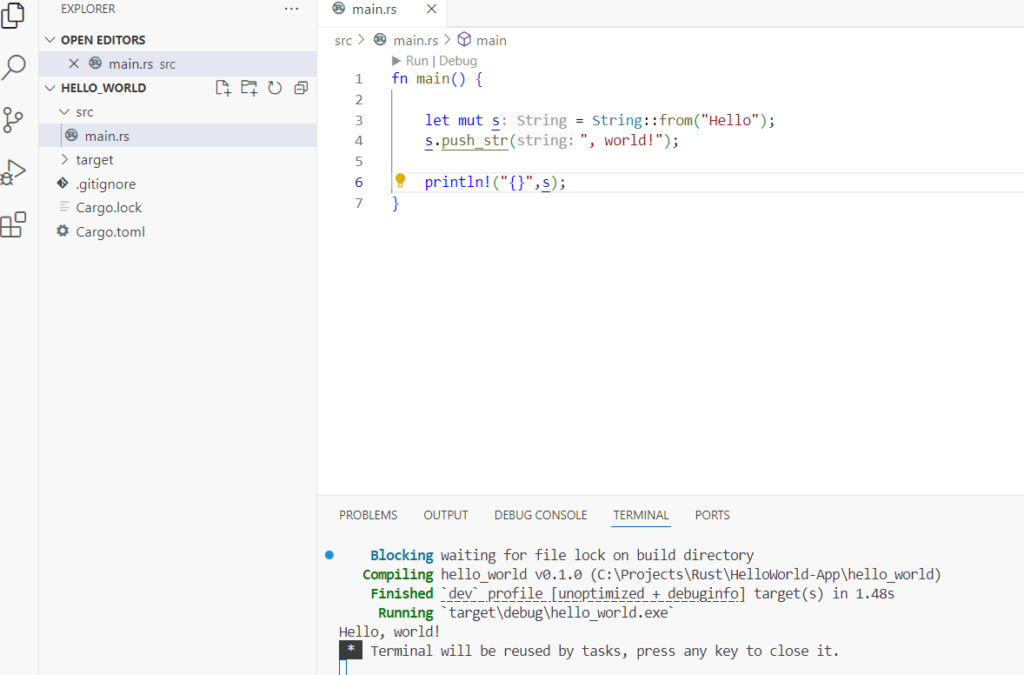
Understanding String Slices
What is a String Slice?
A string slice (&str
) is a reference to a part of a String
. String slices are used to borrow portions of a string without taking ownership.
Creating and Using String Slices
Creating a string slice:
let hello = &s[0..5];
This code creates a slice of the first five characters of s.
HashMaps in Rust
What Are HashMaps?
A HashMap
is a collection that maps keys to values. It is useful for storing data that needs to be quickly retrieved via a key.
Creating and Initializing HashMaps
To create a HashMap:
use std::collections::HashMap;
let mut scores = HashMap::new();
Inserting and Accessing Elements
Inserting elements:
scores.insert(String::from("Blue"), 10);
Accessing elements:
let score = scores.get("Blue");
Iterating Over HashMaps
Iterate over key-value pairs:
for (key, value) in &scores {
println!("{}: {}", key, value);
}
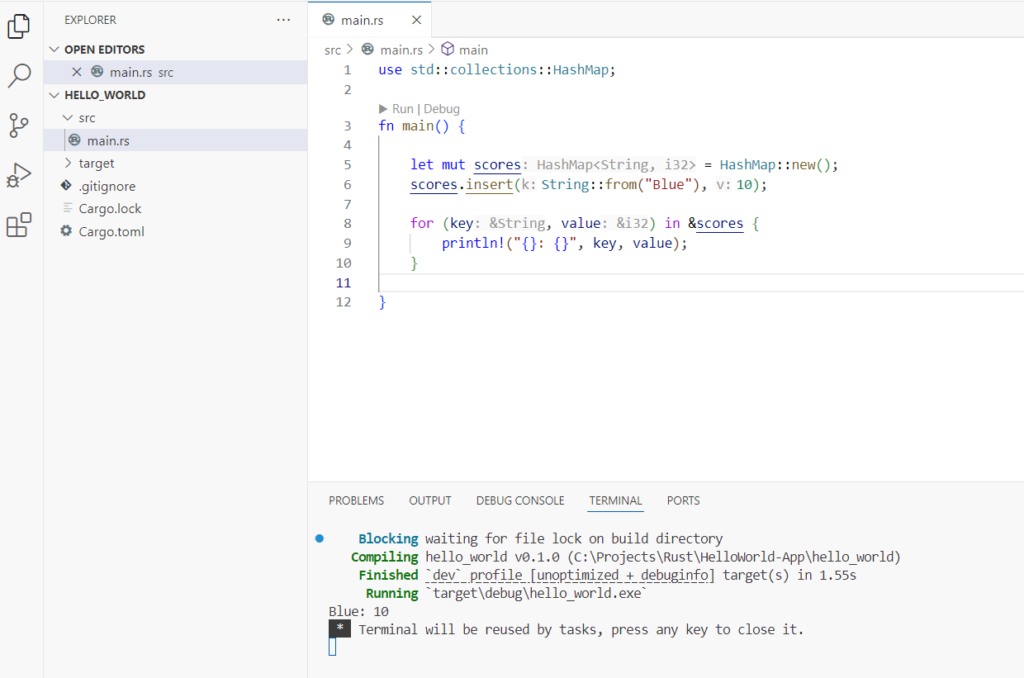
BTreeMap
A BTreeMap
is similar to a HashMap
but maintains keys in sorted order. This is useful when you need ordered data.
HashSet
A HashSet
is a collection of unique values, useful when you want to ensure no duplicates.
Comparison of Collections in Rust
Performance Considerations
- Arrays are the most efficient in terms of memory and speed due to their fixed size.
- Vectors offer flexibility with dynamic sizing but come with a slight performance overhead.
- Strings and String Slices balance flexibility and performance.
- HashMaps provides fast key-value access but may have higher memory usage compared to other collections.
Use Cases
- Arrays: Fixed-size data that won’t change.
- Vectors: Dynamic lists where size can change.
- Strings: Dynamic text management.
- String Slices: Efficient text references.
- HashMaps: Key-value pairs with fast lookup.
Conclusion
Rust’s different ways of storing and organizing data are like different tools for different jobs. You’ve got arrays and vectors for lists of things, strings for text, and HashMaps for pairs of related items. Knowing when to use each one can help you write better code in Rust.
FAQs
1. What is the main difference between arrays and vectors in Rust?
Arrays have a fixed size known at compile time, while vectors can grow or shrink dynamically.
2. How do you choose between a String and a String Slice?
Use String
for owned, mutable text and &str
for borrowed, immutable references to the text.
3. What are the benefits of using HashMaps?
HashMaps provide fast, constant-time complexity for inserting and accessing elements by a key.
4. Can you resize an array in Rust?
No, arrays have a fixed size. Use vectors if you need a resizable collection.
5. How do you iterate over a HashMap?
Use a for
loop to iterate over key-value pairs in the HashMap.
Now you’re equipped with a deeper understanding of collections in Rust. Happy coding!