Contents
Introduction to Error Handling in Rust
In software development, managing errors is an essential part of ensuring the reliability and stability of your applications. Regardless of the programming language, errors are bound to occur, and effectively handling them is crucial. Rust offers robust tools for error handling, prioritizing safety and control when dealing with unexpected situations.
What is Error Handling?
Error handling is a crucial aspect of software development that involves the effective management and response to errors encountered during program execution. These errors can range from basic issues such as file not found errors to more complex like network failures or logical errors within the code itself. Proper error-handling techniques are essential for creating robust and reliable software that can gracefully handle unexpected situations and provide meaningful feedback to users.
Importance of Effective Error Handling
Effective error handling not only prevents crashes but also improves the overall user experience by providing meaningful feedback when something goes wrong. It allows developers to analyse and fix issues fast, ensuring smoother operation of the software.
Error Handling with Result
In Rust, the Result
type is central to error handling. It represents either a successful value (Ok
) or an error (Err
). This explicit handling of errors forces developers to confront and handle potential failures, promoting more reliable code.
Understanding the Result Enum
The Result
enum is defined as:
enum Result<T, E> {
Ok(T),
Err(E),
}
Here, T
represents the type of successful value, and E represents the type of error.
Using Result for Error Propagation
To propagate errors in Rust, functions can return Result<T, E>
where T
is the type of the successful value and E
is the type of error. This allows errors to be passed up through the call stack until they are handled appropriately.
Unwrapping Errors
In Rust, the unwrap()
method is used to extract the value from an Ok
variant or to panic if it encounters an Err
variant. While convenient for prototyping or when you are sure that an error cannot occur, it can lead to crashes if used improperly.
The unwrap() Method
let result: Result<i32, &str> = Ok(42);
let value = result.unwrap(); // Returns 42
let result: Result<i32, &str> = Err("Error message");
let value = result.unwrap(); // Panics with "Error message"
When to Use unwrap()
It’s essential to use unwrap()
judiciously, especially in production code where unexpected errors could occur. Always consider fallback mechanisms or proper error handling instead of relying solely on unwrap()
.
Propagating Errors
Rust provides the ?
operator as a concise way to propagate errors from a function that returns Result
without writing explicit match statements. This operator simplifies error-handling code and improves readability.
Using the ? Operator
fn read_file() -> Result<String, io::Error> {
let mut file = File::open("file.txt")?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
Ok(contents)
}
Benefits of Error Propagation
Error propagation with ?
reduces boilerplate code and ensures that errors are handled consistently across different parts of the codebase. It promotes cleaner, more maintainable Rust code.
Custom Error Types
Custom error types in Rust enable developers to create their error structures, which can offer more precise and context-specific details about failures. This approach improves error handling by customizing error messages and behaviour to better suit the unique requirements of the application.
Creating Custom Error Structs
use std::error::Error;
use std::fmt;
#[derive(Debug)]
struct CustomError {
message: String,
}
impl fmt::Display for CustomError {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
write!(f, "{}", self.message)
}
}
impl Error for CustomError {}
Implementing Error Traits
By implementing the std::error::Error
trait for custom error types, Rust ensures that these errors can be handled uniformly across the application. This includes methods for retrieving error messages and nested errors.
Error Handling Best Practices
Effective error handling goes beyond just implementing mechanisms; it involves adopting best practices to ensure comprehensive coverage of potential error scenarios and seamless recovery from failures.
Handling Different Error Scenarios
It is important to identify and categorize potential error scenarios at an early stage of the development process. It is also crucial to create error-handling strategies that can handle various types of errors, such as unexpected inputs, network failures, and system errors.
Logging and Error Reporting
Implement logging mechanisms to record errors and relevant contextual information. Logging helps in diagnosing issues post-deployment and provides insights into the root causes of errors.
Real-world example
use std::fs::File;
use std::io::{self, Read};
use std::num::ParseIntError;
// Function to read a file's content
fn read_file_content(file_path: &str) -> Result<String, io::Error> {
let mut file = File::open(file_path)?;
let mut content = String::new();
file.read_to_string(&mut content)?;
Ok(content)
}
// Function to parse an integer from a string
fn parse_integer(input: &str) -> Result<i32, ParseIntError> {
input.trim().parse::<i32>()
}
// Function demonstrating multiple error types with custom error type
#[derive(Debug)]
enum CustomError {
IoError(io::Error),
ParseError(ParseIntError),
}
impl From<io::Error> for CustomError {
fn from(error: io::Error) -> Self {
CustomError::IoError(error)
}
}
impl From<ParseIntError> for CustomError {
fn from(error: ParseIntError) -> Self {
CustomError::ParseError(error)
}
}
fn process_file(file_path: &str) -> Result<i32, CustomError> {
let content = read_file_content(file_path)?;
let number = parse_integer(&content)?;
Ok(number)
}
fn main() {
let file_path = "numbers.txt";
// Using match for detailed error handling
match read_file_content(file_path) {
Ok(content) => println!("File content: {}", content),
Err(e) => eprintln!("Failed to read file: {}", e),
}
// Using unwrap_or for default value
let number_str = "42";
let number: i32 = parse_integer(number_str).unwrap_or(0);
println!("Parsed number: {}", number);
// Using unwrap_or_else for custom error handling
let another_number_str = "not_a_number";
let another_number: i32 = parse_integer(another_number_str).unwrap_or_else(|e| {
eprintln!("Failed to parse integer: {}", e);
0
});
println!("Another parsed number: {}", another_number);
// Handling custom error type
match process_file(file_path) {
Ok(number) => println!("Processed number: {}", number),
Err(e) => match e {
CustomError::IoError(io_err) => eprintln!("IO error: {}", io_err),
CustomError::ParseError(parse_err) => eprintln!("Parse error: {}", parse_err),
},
}
}
Result
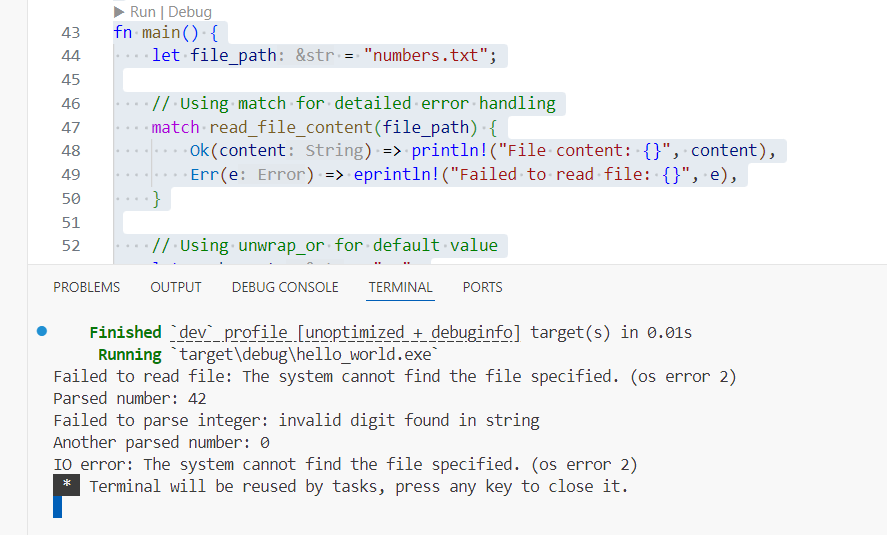
Conclusion
In conclusion, error handling in Rust is designed to promote safety and reliability in software development. By leveraging features like the Result
type, unwrap()
method, ?
operator, and custom error types, developers can build more resilient applications that gracefully handle errors and provide meaningful feedback to users.
Frequently Asked Questions
What is the Result type in Rust?
The Result
type in Rust represents either a successful value (Ok
) or an error (Err
).
When should I use unwrap() in Rust?
unwrap()
should be used cautiously, primarily for prototyping or situations where an error is known to be impossible.
How do I define custom error types in Rust?
Custom error types in Rust are defined by creating structs that implement the std::error::Error
trait.
What are the advantages of using the ? operator for error handling in Rust?
The ?
operator simplifies error propagation, reducing boilerplate code and improving code readability.
How can logging improve error handling in Rust programs?
Logging allows developers to track and diagnose errors in production environments, aiding in troubleshooting and improving overall system reliability.
This concludes the article on Rust Programming Error Handling. I hope you find it informative and useful!