As a Rust programmer, it’s important to understand modules and packages, which are key for organizing code, managing dependencies, and building strong applications. This guide will explain Rust modules, packages, and crates in a way that’s easy to understand and friendly.
Table of Contents
What is a Rust Module?
In Rust, modules are a fundamental building block for organizing code. They serve two main purposes:
- Code Organization: Modules help break down large programs into smaller, logical units. This improves readability and maintainability by grouping related functions, structs, and other code elements.
- Privacy Control: Modules provide a mechanism to control the visibility of code. By default, items within a module are private, meaning they can only be accessed from within the same module. You can explicitly make items public using the
pub
keyword, allowing them to be used from other parts of your program.
Creating Your First Module
Basic Syntax and Structure
Creating a module in Rust is straightforward. You define a module using the mod
keyword, followed by the module’s name and a block of code
mod my_module {
// module contents go here
}
Example: Creating a Simple Module
Let’s create a simple module called greetings
:
mod greetings {
pub fn say_hello() {
println!("Hello, world!");
}
}
Here, pub
makes the function say_hello
public so that it can be accessed outside the module.
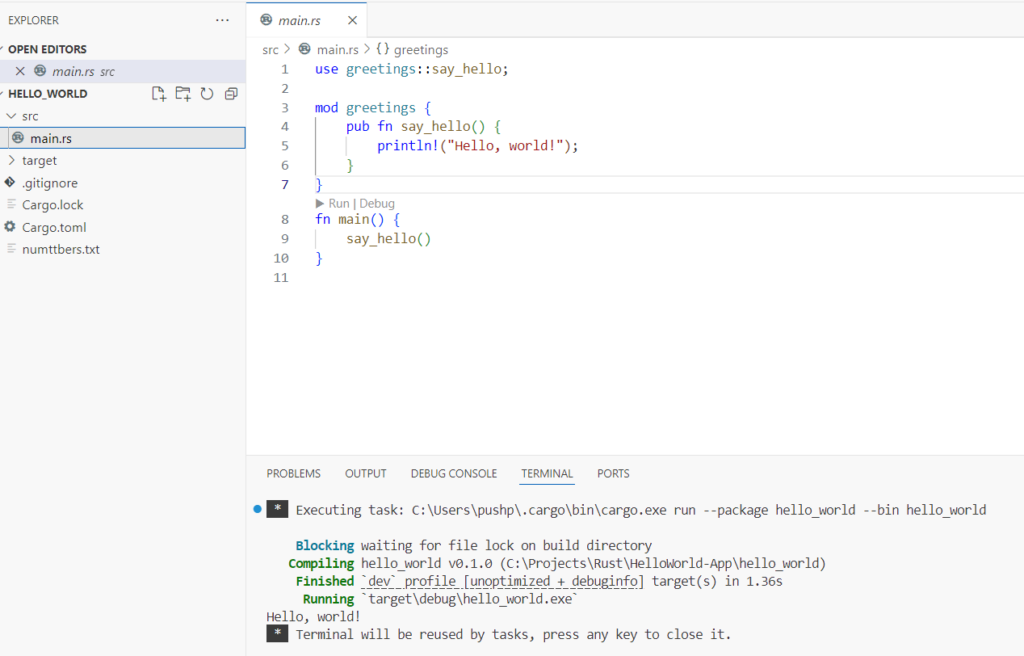
Module Privacy in Rust
Public vs Private Modules
By default, everything in a module is private. This means you can’t access it from outside the module unless you explicitly make it public using the pub
keyword.
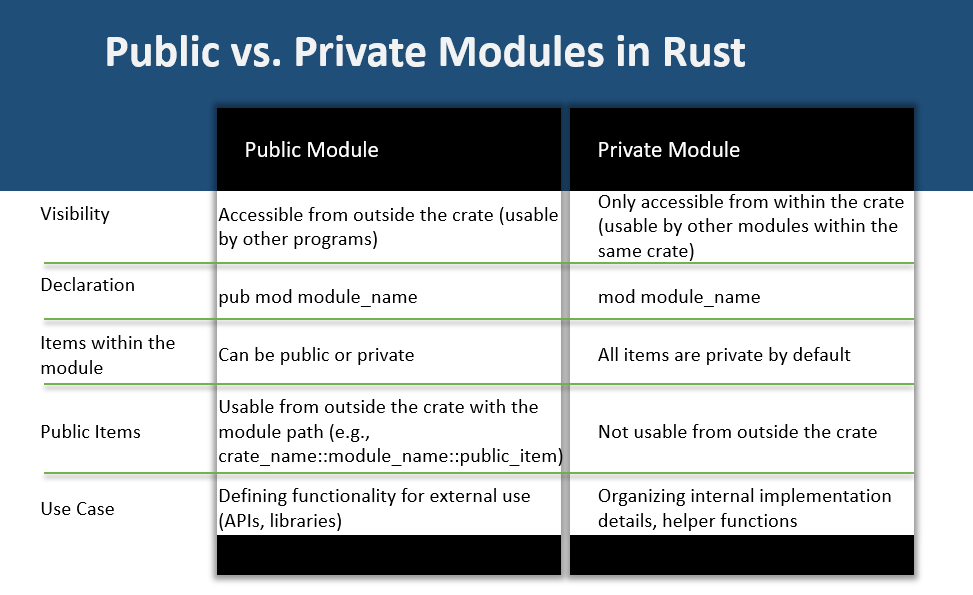
Controlling Access with pub
Keyword
Use the pub
keyword to control the visibility of your modules and their contents. For example:
pub mod public_module {
pub fn public_function() {
println!("This function is public!");
}
}
mod private_module {
pub fn private_function() {
println!("This function is private!");
}
}
What is a Rust Package?
A package in Rust is a collection of one or more crates. It includes a Cargo.toml
file that describes the package’s dependencies, configuration, and metadata.
Creating Packages in Rust
Using Cargo to Create a Package
Cargo is Rust’s package manager and builds the system. To create a new package, use the following command:
cargo new my_package
This command creates a new directory with the package’s name, containing the necessary files and folders.
Understanding Crates in Rust
Binary Crates vs Library Crates
Crates come in two flavours: binary and library. Binary crates are executables, while library crates provide functionality to other crates.
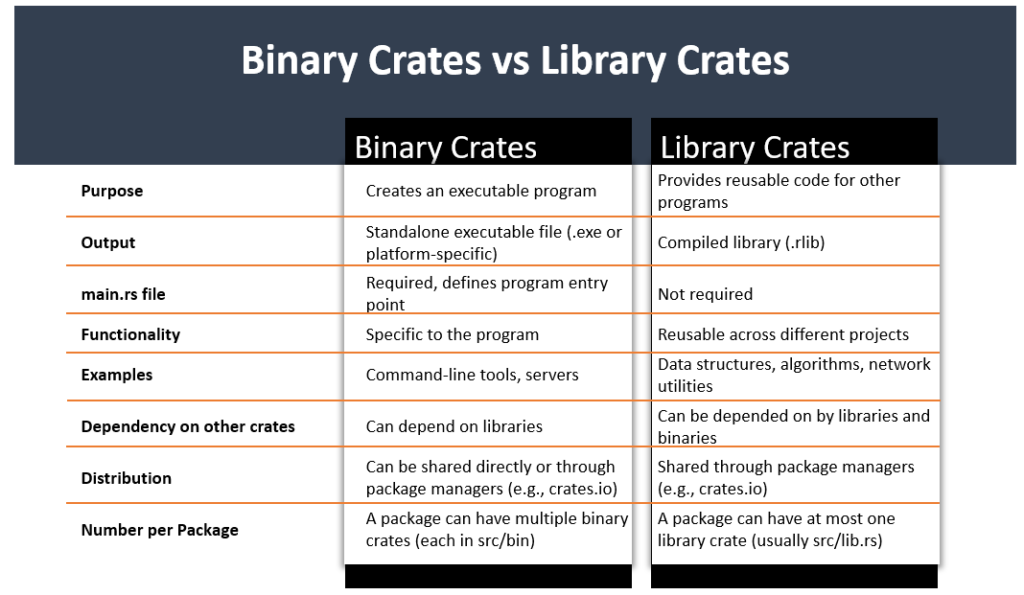
How Crates Work Within Packages
A package can contain multiple crates, but it usually contains one primary crate, either a binary or a library.
Using External Crates
Adding External Crates to Your Project
Finding and Selecting Crates
To add external crates, first, find a crate on crates.io. Look for crates that are well-documented and have good community support.
Adding Dependencies in Cargo.toml
Once you’ve found a crate, add it to your Cargo.toml
file:
[dependencies]
serde = "1.0"
Managing Dependencies
Version Control and Updating Crates
Keeping your dependencies up-to-date is crucial. Specify the version in Cargo.toml
and use cargo update
to update them.
Resolving Dependency Conflicts
Sometimes, different crates might require different versions of the same dependency. Rust handles this with feature flags and careful dependency resolution.
Practical Examples
Building a Simple Application with Modules and Packages
Let’s build a simple app that uses our greetings
module and an external crate:
Create the Project:
cargo new my_app
cd my_app
Add the greetings
Module
// src/lib.rs
pub mod greetings {
pub fn say_hello() {
println!("Hello, world!");
}
}
Add an External Crate:
[dependencies]
chrono = "0.4"
Use the Module and Crate:
// src/main.rs
use my_app::greetings;
use chrono::Local;
fn main() {
greetings::say_hello();
println!("The current time is {}", Local::now());
}
Integrating External Crates for Added Functionality
Crates like serde
for serialization, regex
for regular expressions, and tokio
for asynchronous programming can significantly enhance your Rust projects.
Conclusion
In Rust, modules and packages play an important role in organizing code and facilitating scalability. A module is a collection of items, such as functions, structs, and traits, while a package is a collection of one or more crates. Understanding how to structure and manage modules within a package is essential for writing clean and efficient Rust code. Furthermore, mastering these concepts enables developers to build complex and scalable Rust applications with ease. By leveraging the power of Rust modules and packages, developers can improve code organization, promote reusability, and effectively manage large codebases.
FAQs
What are the benefits of using modules in Rust?
Modules help organize your code, make it more readable, and manage namespaces effectively.
How do you make a module public in Rust?
Use the pub
keyword before the module or its contents to make them public.
What is the difference between a package and a crate in Rust?
A package is a collection of crates, managed by Cargo. A crate is a compilation unit, either a library or a binary.
How can I find external crates for my project?
Visit crates.io to search for and evaluate crates based on documentation, community support, and usage.
What should I do if I encounter a dependency conflict?
Use feature flags and carefully manage versions in Cargo.toml
. Sometimes, updating or downgrading dependencies can resolve conflicts.
I was just as enthralled by your work as you were. Your sketch is elegant, and your written content is sophisticated. However, you seem concerned about potentially delivering something questionable soon. I’m confident you’ll resolve this issue quickly and return to your usual high standards.
Your work has captivated me just as much as it has captivated you. The visual presentation is elegant, and the written content is sophisticated. However, you appear concerned about the possibility of presenting something that could be considered dubious. I’m confident you’ll be able to resolve this issue promptly.
I loved as much as you will receive carried out right here The sketch is tasteful your authored subject matter stylish nonetheless you command get got an edginess over that you wish be delivering the following unwell unquestionably come further formerly again as exactly the same nearly very often inside case you shield this hike
Thank you I have just been searching for information approximately this topic for a while and yours is the best I have found out so far However what in regards to the bottom line Are you certain concerning the supply
I was recommended this website by my cousin I am not sure whether this post is written by him as nobody else know such detailed about my difficulty You are wonderful Thanks
Your blog is a beacon of light in the often murky waters of online content. Your thoughtful analysis and insightful commentary never fail to leave a lasting impression. Keep up the amazing work!
Its like you read my mind You appear to know so much about this like you wrote the book in it or something I think that you can do with a few pics to drive the message home a little bit but instead of that this is excellent blog A fantastic read Ill certainly be back
Nice blog here Also your site loads up very fast What host are you using Can I get your affiliate link to your host I wish my site loaded up as quickly as yours lol
Usually I do not read article on blogs however I would like to say that this writeup very compelled me to take a look at and do it Your writing style has been amazed me Thank you very nice article
you are in reality a just right webmaster The site loading velocity is incredible It seems that you are doing any unique trick In addition The contents are masterwork you have performed a wonderful task on this topic
Comments are closed.