Contents
Introduction
Rust is a high-performance programming language known for its focus on safety. One of its key features revolves around the use of structs and enums. These concepts are essential to fully leverage the power of Rust. In this complete article, we will explore in-depth the process of defining and using structs, method syntax, enums, and pattern matching. We will also delve into the practical applications of the Option and Result enums.
Importance of Structs and Enums
Structs and enums are fundamental to Rust programming. They allow you to create complex data types that are easy to manage and use, making your code more readable and maintainable.
Defining and Using Structs
What is a Struct?
In Rust, a struct is a custom data type that lets you name and package together multiple related values. It is a way to group related variables, similar to a class in object-oriented languages, but without methods.
Defining Structs
- Keyword
struct
: You use thestruct
keyword to declare a new struct. - Struct Name: Choose a descriptive name in UpperCamelCase (e.g.,
Point2D
,UserInfo
). - Fields: Inside curly braces
{}
, define the fields of the struct. Each field has a name and a data type (e.g., string, integer).
Types of Structs
Tuple Structs
Tuple structs are similar to tuples but are used when you want to give the whole tuple a name and access its elements by position.
struct Color(u8, u8, u8);
Named Field Structs
These structs have named fields, making it clear what each data point represents.
struct Point {
x: f32,
y: f32,
}
Unit Structs
Unit structs are used when you need to implement a trait on a type but don’t need to store any data.
struct AlwaysEqual;
Creating Structs in Rust
struct Rectangle {
width: u32,
height: u32,
}
let rectangle = Rectangle {
width: 35,
height: 50,
};
println!("Rectangle width: {}, height: {}", rectangle.width, rectangle.height);
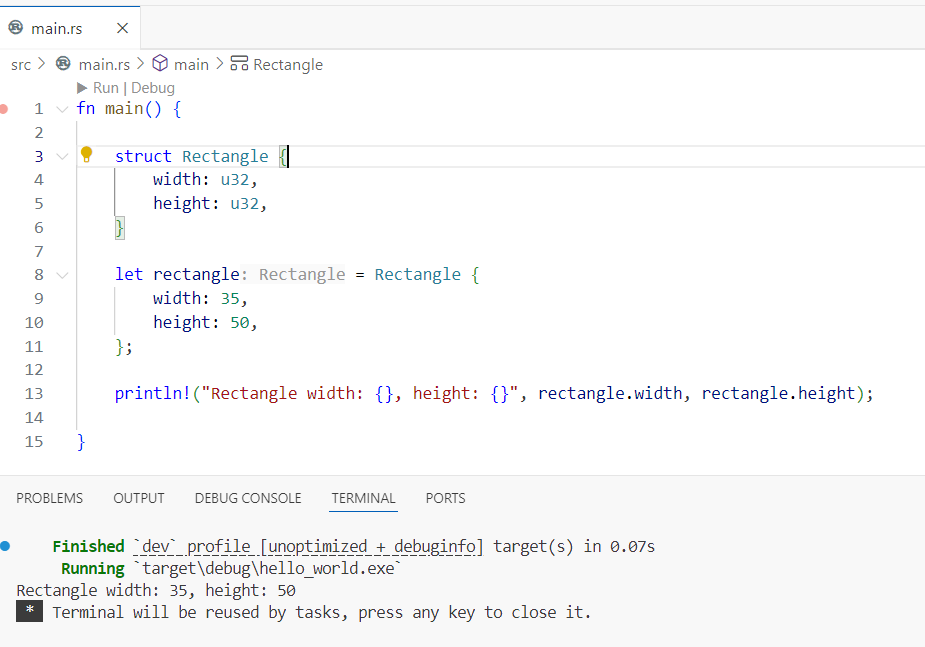
Method Syntax
Understanding Method Syntax in Rust
Methods are functions defined within the context of a struct, enum, or trait object. They are used to perform operations on the data contained within these types.
Defining Methods:
impl
Block: You define methods within animpl
block associated with a specific type (struct or enum). This block tells Rust which type the methods belong to.self
Parameter: The first parameter of a method is always namedself
. It refers to the instance of the struct or enum that the method is being called on. This gives the method access to the data stored within the instance.
Implementing Methods for Structs
Associated Functions
Associated functions are functions that are associated with a struct but don’t take self as a parameter.
impl Rectangle {
fn square(size: u32) -> Rectangle {
Rectangle { width: size, height: size }
}
}
Methods with &self
Methods that take &self as a parameter borrow the instance of the struct they are called on.
impl Rectangle {
fn area(&self) -> u32 {
self.width * self.height
}
}
Example: Adding Methods to a Struct
Let’s add some methods to our Rectangle struct.
impl Rectangle {
fn area(&self) -> u32 {
self.width * self.height
}
fn can_hold(&self, other: &Rectangle) -> bool {
self.width > other.width && self.height > other.height
}
}
let rect1 = Rectangle {
width: 30,
height: 50,
};
let rect2 = Rectangle {
width: 10,
height: 40,
};
println!("The area of rect1 is: {}", rect1.area());
println!("Can rect1 hold rect2? {}", rect1.can_hold(&rect2));
Enums and Pattern Matching
What is an Enum?
An enum is a type that can represent one of several variants. It’s a powerful way to handle different kinds of data under a single type.
Defining Enums in Rust
Enums are defined using the enum keyword.
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
ChangeColor(i32, i32, i32),
}
Pattern Matching with Enums
Pattern matching allows you to compare a value against a series of patterns and execute code based on which pattern matches.
The match Control Flow Operator
The match operator is used to handle different enum variants.
fn process_message(msg: Message) {
match msg {
Message::Quit => println!("Quit"),
Message::Move { x, y } => println!("Move to ({}, {})", x, y),
Message::Write(text) => println!("Text: {}", text),
Message::ChangeColor(r, g, b) => println!("Change color to ({}, {}, {})", r, g, b),
}
}
Here’s an example demonstrating enums and pattern matching.
enum IpAddr {
V4(String),
V6(String),
}
fn display_ip(ip: IpAddr) {
match ip {
IpAddr::V4(addr) => println!("IPv4 address: {}", addr),
IpAddr::V6(addr) => println!("IPv6 address: {}", addr),
}
}
let home = IpAddr::V4(String::from("127.0.0.1"));
let loopback = IpAddr::V6(String::from("::1"));
display_ip(home);
display_ip(loopback);
Using the Option and Result Enums
Introduction to Option and Result
Option and Result are enums provided by the standard library to handle scenarios where data might be missing or operations might fail.
The Option Enum
The Option enum is used for optional values, indicating a value can be either Some(T) or None.
Some and None Variants
let some_number = Some(5);
let absent_number: Option<i32> = None;
The Result Enum
The Result enum is used for error handling and contains Ok(T) and Err(E).
Ok and Err Variants
enum Result<T, E> {
Ok(T),
Err(E),
}
fn divide(a: f64, b: f64) -> Result<f64, String> {
if b == 0.0 {
Err(String::from("Cannot divide by zero"))
} else {
Ok(a / b)
}
}
Let’s look at a practical example.
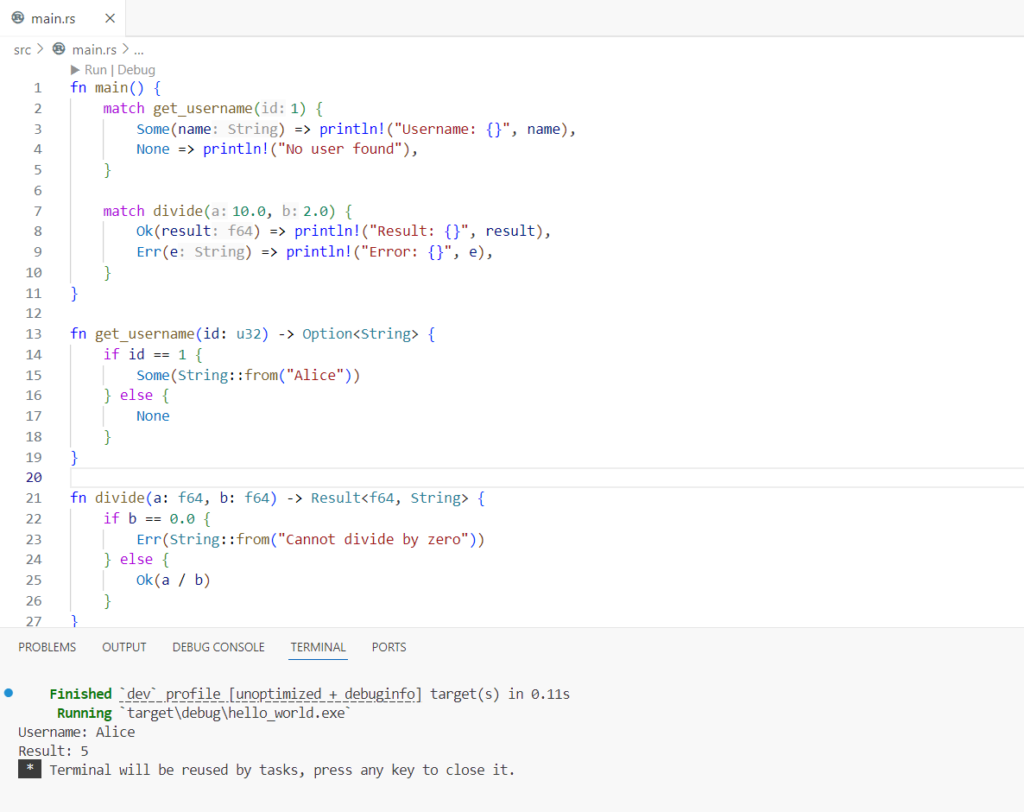
If you try to divide by zero
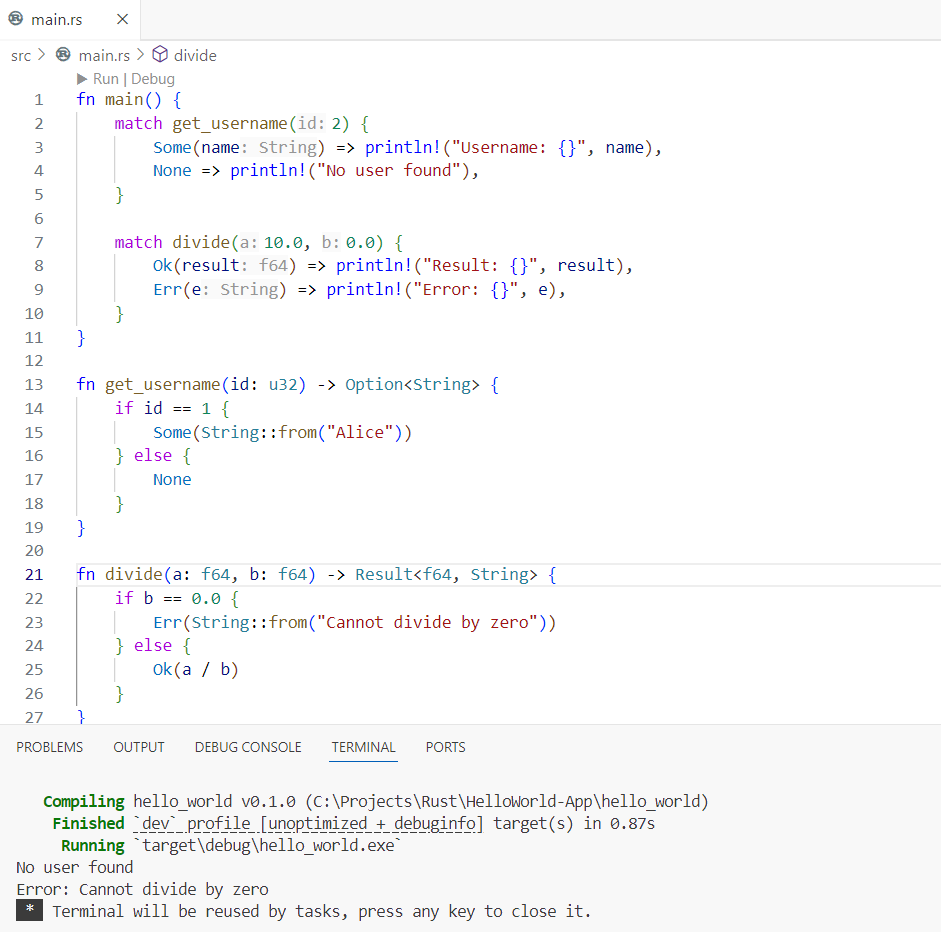
Conclusion
Structs and enums are important building blocks in Rust programming. They provide useful ways to organize and work with data effectively. By learning about and using these tools, you can write Rust code that is strong, easy to read, and simple to maintain. Whether you’re organizing data structurally or managing different options with enums, getting the hang of these concepts will improve your Rust programming abilities.
FAQs
What are the key differences between structs and enums?
Structs group together multiple related data, while enums allow a variable to be one of several different types.
How does pattern matching improve code readability?
Pattern matching lets you concisely handle different cases, making your code easier to read and maintain.
Can I use structs within enums and vice versa?
Yes, structs can be variants in enums, and enums can be fields within structs, providing flexible and powerful data representations.
What are some best practices for defining methods in structs?
Use &self for methods that don’t modify the struct, &mut self for methods that do, and associated functions for functionality related to the struct but not tied to an instance.
How do Option and Result enums help in error handling?
They provide a clear and explicit way to handle the potential absence of data (Option) or the success/failure of operations (Result), making your code more robust and error-resistant.