Rust is an extremely fast programming language that supports speed, concurrency, as well as multithreading. In Rust, we have a concept of Traits and Generics where Generics are an abstraction of various properties, and Traits are used for combining generic types for constraining types for accepting particular behaviour of various types.
Contents
Real-Life Examples of Traits and Generics
Let’s say you have a Printer
trait. It might have a print()
method. Now, you can implement this trait for a Book
, Document
, or even a Spreadsheet
. All you need to know is that these types have a print()
method—they might do it differently, but as long as they stick to the contract, you’re good to go.
Defining and Implementing Traits
trait Printer {
fn print(&self);
}
Implementing Traits in Rust
struct Book {
title: String,
}
impl Printer for Book {
fn print(&self) {
println!("Printing book: {}", self.title);
}
}
- This defines a structure named
Book
.It has a single field,title
, which is of typeString
. This field will store the title of the book. - This block implements the
Printer
trait for theBook
structure. - Traits in Rust are similar to interfaces in other languages. They define a set of methods that a type must implement to be considered compatible with that trait.
- The
Printer
trait (which we assume is defined elsewhere) likely has aprint
method, and this implementation provides the specific behavior for theBook
type. - The
print
method takes a reference to theBook
instance (&self
) and prints the book’s title to the console usingprintln!
.
Combining Trait Bounds and Generics
fn print_item<T: Printer>(item: T) {
item.print();
}
- The
print_item
function provides a generic way to print any item that implements thePrinter
trait. - This means you can use the same function to print different types of objects, as long as they have a
print
method that follows thePrinter
trait’s definition.
Output
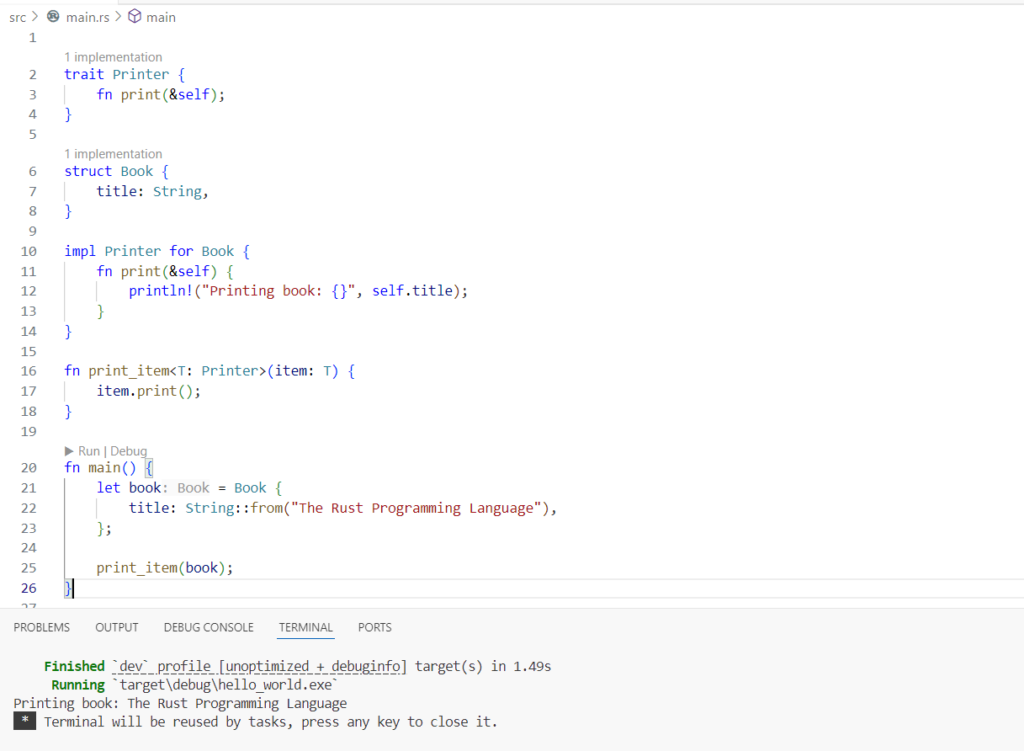
I am truly thankful to the owner of this web site who has shared this fantastic piece of writing at at this place.
hiI like your writing so much share we be in contact more approximately your article on AOL I need a specialist in this area to resolve my problem Maybe that is you Looking ahead to see you
Attractive section of content I just stumbled upon your blog and in accession capital to assert that I get actually enjoyed account your blog posts Anyway I will be subscribing to your augment and even I achievement you access consistently fast
Pretty! This has been a really wonderful post. Many thanks for providing these details.
I appreciate you sharing this blog post. Thanks Again. Cool.
Attractive section of content I just stumbled upon your blog and in accession capital to assert that I get actually enjoyed account your blog posts Anyway I will be subscribing to your augment and even I achievement you access consistently fast
helloI really like your writing so a lot share we keep up a correspondence extra approximately your post on AOL I need an expert in this house to unravel my problem May be that is you Taking a look ahead to see you
I just could not depart your web site prior to suggesting that I really loved the usual info an individual supply in your visitors Is gonna be back regularly to check up on new posts
Comments are closed.