Contents
Understanding Smart Pointers
Smart pointers in Rust are one of the most powerful memory management tools you will see. If you have ever been frustrated by manually managing memory, you will love smart pointers’ efficiency and control. They allow for the attainment of memory safety while still being able to provide flexibility. In this article, we’ll cover the different types of smart pointers. We’re going to be learning about Box, Rc, and RefCell; also, weak references and how those make Rust all that unique when thinking about memory management.
What are Smart Pointers?
Explain in simple form: smart pointers are not just pointers. Yes, they point to data, but they bring a lot of baggage along with them, like reference counting. Just as regular pointers themselves are basically super-powered – as they don’t just hold addresses but actually manage the memory they point to.
Why Use Smart Pointers?
Memory management gets very tricky and complicated, especially in greater systems. This is the point where smart pointers come in handy.
Efficiency in Memory Management
The smart pointer automatically manages the memory and cleans up after itself when no longer needed. It saves overhead, you don’t need to free the allocated memory as a smart pointer would do this for you.
Preventing Memory Leaks
Smart pointers in Rust avoid memory leaks through either automatic reference counting or unique ownership principles. You no longer have to worry about portions of memory being left behind, unused and taking up valuable resources in your system.
Types of Smart Pointers in Rust
Let’s see three of the most important types of smart pointers that Rust has to offer: Box, Rc, and RefCell. Each of them serves a specific purpose in memory management to help you allocate and manage data safely and efficiently.
Box Smart Pointer
What is a Box?
Box smart pointer in Rust will let you save data on the heap rather than the stack. The stack is fast and efficient when dealing with little data. However, with complex or large data structures, the heap is better suited for them. Box helps you do just that.
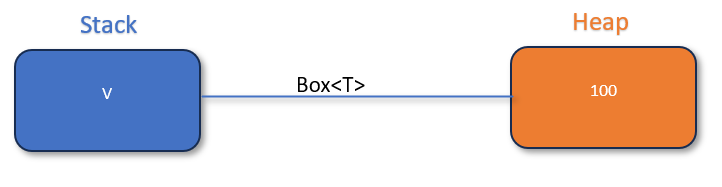
How Box Works?
The box creates heap-allocated data and then points to that. It allows you to use this data as if it were on the stack. This is primarily useful when working with recursive data types or wanting to allocate large data.
Box and Memory Allocation
Once the Box goes out of scope, Rust automatically deallocates the memory on the heap. Simple and efficient, right? It’s a safe way to handle heap data without manual memory management.
use std::boxed::Box;
fn main() {
// Create a Box on the heap
let box_value: Box<i32> = Box::new(42);
// Access the value through dereferencing
println!("Value: {}", *box_value);
// Move the ownership of the Box to another variable
let another_box = box_value;
// The original box_value is now invalid
// println!("Invalid value: {}", *box_value); // This would cause a compile-time error
// Access the value through the new box
println!("Another value: {}", *another_box);
// Explicitly drop the Box to free the memory
drop(another_box);
// Now the memory is deallocated
}
Rc Smart Pointer
What is Rc?
Rc stands for Reference Counting. Rc will allow more than one part of your code to own a piece of data. You will have shared ownership with Rc, which is ideal for scenarios where more than one entity should either read or have the same data in their possession.
Shared Ownership with Rc
Rc comes into good stead when different parts of the code need to have access to data without taking ownership of it. It will keep count of how many references to the data are in existence, and ensure the memory is released only after all the references are done with it.
Reference Counting – Rc
For every clone of an Rc, the reference count is increased, and it’s dropped when the count goes down. When the count reaches zero, it frees the memory. Thus, automatic reference counting prevents memory leaks from happening.
use std::rc::Rc;
fn main() {
// Create an Rc pointing to a string
let rc_str = Rc::new("Hello, world!");
// Clone the Rc to create a second reference
let rc_str_clone = rc_str.clone();
// Print the string through both references
println!("String 1: {}", rc_str);
println!("String 2: {}", rc_str_clone);
// The string is shared between both references
// Modifying one would affect the other
// Drop one of the references
drop(rc_str_clone);
// The string is still accessible through the remaining reference
println!("String 1 (after dropping): {}", rc_str);
// The string will be dropped when the last Rc reference is dropped
}
RefCell Smart Pointer
What is RefCell?
RefCell allows a mutable memory location inside an immutable data structure. Yes, you heard that right. It provides the capability of modifying data even when it’s actually immutable outside.
Interior Mutability Explained
The major power of RefCell is a feature called interior mutability. It provides mutable access to data even when that data is otherwise immutably borrowed. It’s like giving your program the superpower to break the rules but in a very controlled way!
But why does RefCell have such a special status?
RefCell does runtime borrow checking, whereas Rust normally does borrow checking at compile time. This means that RefCell has a bit more flexibility but also comes with some minor performance penalties.
use std::cell::RefCell;
fn main() {
// Create a RefCell containing a mutable integer
let refcell_value = RefCell::new(42);
// Access the value through borrowing
let borrowed_value = refcell_value.borrow();
println!("Borrowed value: {}", borrowed_value);
// Modify the value through a mutable borrow
let mut borrowed_value_mut = refcell_value.borrow_mut();
*borrowed_value_mut += 10;
println!("Modified value: {}", borrowed_value_mut);
// Access the value again through a shared borrow
let borrowed_value = refcell_value.borrow();
println!("Borrowed value (after modification): {}", borrowed_value);
// The RefCell prevents multiple mutable borrows at the same time
// let mut another_borrow_mut = refcell_value.borrow_mut(); // This would cause a compile-time error
}
Weak References in Rust
In Rust, weak references extend Rc to offer non-owning references to data. While Rc handles shared ownership, Weak will prevent problems of reference cycles from occurring.
What Are Weak References?
The Weak reference allows you to refer to data without owning the data. This enables a kind of “backup pointer” which does not own anything, hence preventing any potential reference cycles.
Differences Between Rc and Weak References
Unlike Rc, a Weak reference isn’t an owner to the data it points to. So even though they can’t prevent data from being dropped, they also can’t cause data to leak. Additionally, this means a cycle of Rc s will prevent the data they point to from being dropped. Also, a Weak reference is used to create a reference cycle where using Rc would create a reference cycle.
When to Use Weak References?
This is quite a good solution where you want to avoid reference cycles, particularly those instances where data may refer back to each other. It’s a safer way of avoiding memory leaks across these cyclical relationships.
use std::cell::RefCell;
use std::rc::{Rc, Weak};
struct Node {
value: i32,
next: RefCell<Option<Weak<Node>>>,
}
fn main() {
let node1 = Rc::new(Node { value: 1, next: RefCell::new(None) });
let node2 = Rc::new(Node { value: 2, next: RefCell::new(None) });
// Connect the nodes
node1.next.borrow_mut().replace(Rc::downgrade(&node2));
node2.next.borrow_mut().replace(Rc::downgrade(&node1));
// Print the linked list
let mut current = Some(node1.clone());
while let Some(node) = current {
println!("Value: {}", node.value);
current = node.next.borrow().as_ref().and_then(|weak| weak.upgrade());
}
// Drop the strong references
drop(node1);
drop(node2);
// The weak references are now invalid
}
Memory Management Concepts in Rust
Rust provides a robust and tightly coupled memory management system based on ownership and borrowing principles. These concepts are crucial in as far as making sure that memory is used in an efficient and secure manner.
Ownership and Borrowing in Rust
Ownership Rules
Rust enforces strict rules about ownership. Each value in Rust has a variable that is considered to be its owner. There can only be one owner at a time. This ensures the memory occupying a given value is deallocated exactly once, without leaving any dangling pointers to the memory.
Borrowing and Lifetimes
Instead of ownership, Rust provides with borrowing. You can have references to data without taking ownership of the data. Rust will check these references compile time to ensure they are valid in the right scope.
Stack vs Heap Memory
What Goes on the Stack?
The stack will typically hold simple data types, like integers or small arrays. It’s fast, but limited space, so it’s perfect for small and temporary data.
What Goes on the Heap?
The heap is used for larger data structures, or data that needs to persist after a function has returned. Access via the heap is slower, but far more flexible and roomy, allowing for dynamic allocation.
Garbage Collection vs Manual Memory Management
Rust’s Unique Memory Management Model
Unlike many modern languages, Rust does not use garbage collection. Instead, it uses its unique concepts of ownership and borrowing to manage memory at compile time. This results in greater performance in the absence of a garbage collector.
Benefits of the Model Adopted by Rust
Rust’s model evades the overhead of garbage collection and yet guarantees memory safety. You almost get the best of both worlds, wherein you get efficient usage of memory without sacrificing speed or safety.
Conclusion
Smart pointers include Box, Rc, and RefCell, among others, that facilitate flexibility and power in memory management in Rust. Learning the mechanisms behind such abstractions gives you programs with efficient running, while avoiding common pitfalls like memory leaks. Mastering these tools will give one a firm grasp on Rust’s memory management model and enable him or her to write safer, more efficient code.
Thank you for the auspicious writeup It in fact was a amusement account it Look advanced to more added agreeable from you By the way how could we communicate
Somebody essentially help to make significantly articles Id state This is the first time I frequented your web page and up to now I surprised with the research you made to make this actual post incredible Fantastic job
Simply desire to say your article is as surprising The clearness in your post is simply excellent and i could assume you are an expert on this subject Fine with your permission let me to grab your feed to keep up to date with forthcoming post Thanks a million and please carry on the gratifying work
certainly like your website but you need to take a look at the spelling on quite a few of your posts Many of them are rife with spelling problems and I find it very troublesome to inform the reality nevertheless I will definitely come back again
Thank you for the good writeup It in fact was a amusement account it Look advanced to far added agreeable from you However how could we communicate
Comments are closed.