Contents
After you import following code for the Anjular Service Class “import { HttpClient, HttpHeaders } from ‘@angular/common/http’;”. Almost certainly get the following Angular Error StaticInjectorError (AppModule)[HttpClient]
What is StaticInjectorError?
Angular Error StaticInjectorError is occurs during the process of dependency injection in Angular applications. It arises when the Angular injector cannot resolve a dependency, resulting in a failure to instantiate a component or service.
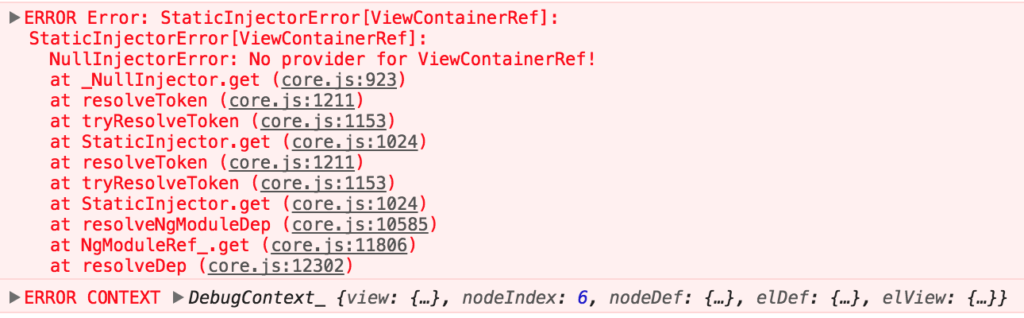
Understanding Dependency Injection
Before delving deeper into Angular Error StaticInjectorError, it’s essential to grasp the concept of dependency injection (DI). DI is a design pattern used to manage the dependencies of an application by providing components with the objects they depend on. In Angular, DI plays a crucial role in facilitating loose coupling and enhancing testability.
Explanation of StaticInjectorError
Definition and Causes StaticInjectorError is triggered when the Angular injector fails to resolve a dependency at compile time. This can occur due to various reasons, including incorrect provider configuration, circular dependencies, or missing imports.
Common Scenarios Triggering StaticInjectorError Some common scenarios leading to StaticInjectorError include:
- Incorrect configuration of providers in modules or components.
- Circular dependencies between services.
- Missing or incorrectly imported modules.
Impact of StaticInjectorError
Consequences on Application Functionality The impact of StaticInjectorError can be profound, causing components or services to fail to instantiate properly. This can lead to runtime errors, application crashes, or unexpected behavior, severely impacting user experience.
Troubleshooting StaticInjectorError
Strategies for Identifying the Root Cause When faced with StaticInjectorError, developers must adopt a systematic approach to identify the root cause. This involves:
- Reviewing error logs and stack traces.
- Inspecting the code for potential misconfigurations.
- Utilizing debugging tools and techniques.
Resolve the Angular Error StaticInjectorError
You need to change the app.module.ts file
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent,
……………………….
],
imports: [
…………. ,
HttpClientModule
],
Conclusion
In conclusion, StaticInjectorError is a common yet perplexing issue encountered in Angular development. By understanding its causes, impacts, and troubleshooting strategies, developers can effectively address this error and ensure the smooth functioning of their applications. By adhering to best practices, optimizing code structure, and leveraging community support and resources, developers can mitigate the risk of StaticInjectorError and deliver high-quality Angular applications.
FAQs (Frequently Asked Questions)
- What is StaticInjectorError in Angular? StaticInjectorError is an error that occurs during the process of dependency injection in Angular applications when the injector fails to resolve a dependency.
- How can I troubleshoot StaticInjectorError? To troubleshoot StaticInjectorError, developers can review error logs, inspect code for misconfigurations, and utilize debugging tools to identify the root cause.
- What are some preventive measures against StaticInjectorError? Preventive measures include properly configuring providers, avoiding circular dependencies, and conducting thorough code reviews.
- Where can I find community support for StaticInjectorError? Developers can seek assistance from online forums and communities such as Stack Overflow, Angular Google Groups, and Reddit’s Angular community.
- How does StaticInjectorError impact development workflow? StaticInjectorError can disrupt development workflow by causing delays in project timelines and deadlines, requiring extensive debugging and troubleshooting efforts.