Contents
In this article we are looking How to access Web API in C# asp.Net server code
In today’s digital age, harnessing the power of web APIs (Application Programming Interfaces) has become a cornerstone of modern software development. For C# ASP.NET developers, integrating web APIs into server-side code opens up a realm of possibilities, from accessing external data sources to enhancing application functionality. In this comprehensive guide, we’ll delve into the intricacies of accessing web APIs in C# ASP.NET server code, empowering you to unlock the full potential of your applications.
Understanding the Basics: What is a Web API?
Before diving into the nitty-gritty of accessing web APIs in C# ASP.NET, let’s first establish a clear understanding of what exactly a web API is. At its core, a web API serves as an intermediary that allows different software applications to communicate with each other. It defines a set of rules and protocols that enable seamless interaction between disparate systems over the internet.
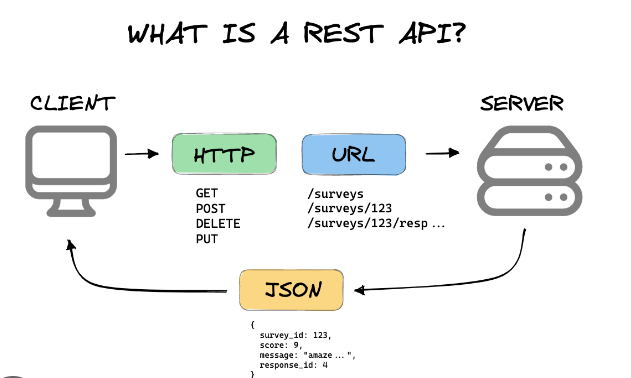
Choosing the Right Tools: Leveraging C# ASP.NET for Web API Integration
When it comes to integrating web APIs into C# ASP.NET server code, having the right tools at your disposal is paramount. Fortunately, the ASP.NET framework provides robust support for building web applications and services, making it an ideal choice for web API integration.
Step-by-Step Guide: Access Web API in C# asp.Net Server Code
Now that we’ve laid the groundwork, let’s walk through a step-by-step guide on how to access web APIs in C# ASP.NET server code. Follow these simple steps to seamlessly integrate external data sources and enhance the functionality of your applications:
- Identify the Target Web API: Before you can access a web API in your C# ASP.NET server code, you’ll need to identify the target API that you wish to integrate. Whether it’s a weather API, social media API, or financial data API, ensure that it aligns with your application’s requirements.
- Authenticate Your Requests: Many web APIs require authentication to access their resources securely. Whether it’s through API keys, OAuth tokens, or other authentication mechanisms, make sure to authenticate your requests properly to avoid unauthorized access.
- Send HTTP Requests: Once authenticated, you can start sending HTTP requests to the web API endpoints to retrieve data or perform actions. In C# ASP.NET, you can use libraries like HttpClient to send GET, POST, PUT, or DELETE requests effortlessly.
- Handle Responses: Upon receiving responses from the web API, it’s essential to handle them appropriately in your C# ASP.NET server code. Whether it’s parsing JSON or XML data, error handling, or processing the results, ensure that your code is robust and resilient.
- Implement Error Handling: Murphy’s Law states that anything that can go wrong will go wrong, so it’s crucial to implement robust error handling mechanisms in your C# ASP.NET code. Whether it’s handling network errors, timeouts, or API rate limits, anticipate potential issues and handle them gracefully.
Best Practices for Web API Integration in C# ASP.NET
As you embark on your journey to master web API integration in C# ASP.NET server code, keep these best practices in mind to ensure smooth sailing:
- Stay Updated: Web APIs are constantly evolving, with new features, updates, and deprecations being introduced regularly. Stay abreast of the latest developments in the APIs you integrate and adjust your code accordingly.
- Optimize Performance: When accessing web APIs in C# ASP.NET server code, performance optimization is key. Minimize latency, reduce network overhead, and implement caching mechanisms to enhance the responsiveness and efficiency of your applications.
- Ensure Security: Security should always be a top priority when integrating web APIs into your C# ASP.NET server code. Implement secure communication protocols, sanitize input data, and adhere to best practices for data protection to safeguard against potential security threats.
Example: Access Web API in C# asp.Net
string res = "";
dynamic json = new JObject();
json.grant_type = "password";
json.username = txtUserName.Text;
json.password = txtPassword.Text;
JObject jObj = JObject.FromObject(json);
var request_json = String.Join("&",jObj.Children().Cast<JProperty>()
.Select(jp => jp.Name + "=" + HttpUtility.UrlEncode(jp.Value.ToString())));
HttpContent content = new StringContent(request_json, Encoding.UTF8, "application/json");
HttpClient client = new HttpClient();
try
{
var response = client.PostAsync("http://localhost:12345/token", content).Result;
if (response.IsSuccessStatusCode)
{
using(HttpContent mMessage = response.Content)
{
res = mMessage.ReadAsAsync<UserAuthToken>().Result.access_token;
}
}
}
catch (Exception mMsg)
{
return mMsg.Message.ToString();
}
return res;
public class UserAuthToken
{
public string access_token { get; set; }
public string token_type { get; set; }
public string expires_in { get; set; }
}
Conclusion
In conclusion, mastering the art of Access Web API in C# asp.Net server code opens up a world of possibilities for developers. By following the steps outlined in this guide and adhering to best practices, you can seamlessly integrate external data sources, enhance application functionality, and deliver exceptional user experiences. So, roll up your sleeves, dive into the world of web API integration, and unlock the full potential of your C# ASP.NET applications.