Contents
In the C# .NET development, harnessing cutting-edge technologies is paramount to stay ahead of the curve. One such technology that has been making waves is gRPC, a high-performance, open-source framework. In this comprehensive guide, we’ll delve into the world of gRPC, exploring its features and implementation in C# gRPC Example.
What is gRPC ?
In gRPC, a client application can directly call a method on a server application on a different machine as if it were a local object, making it easier for you to create distributed applications and services. As in many RPC systems, gRPC is based around the idea of defining a service, specifying the methods that can be called remotely with their parameters and return types. On the server side, the server implements this interface and runs a gRPC server to handle client calls. On the client side, the client has a stub (referred to as just a client in some languages) that provides the same methods as the server. [https://grpc.io/docs/what-is-grpc/]
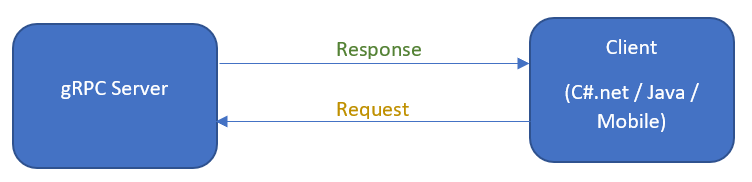
Why gRPC ?
gRPC is a modern open-source high performance Remote Procedure Call (RPC) framework that can run in any environment. It can efficiently connect services in and across data centers with pluggable support for load balancing, tracing, health checking and authentication. It is also applicable in last mile of distributed computing to connect devices, mobile applications and browsers to backend services.
gRPC clients and servers can run and talk to each other in a variety of environments – from servers inside Google to your own desktop – and can be written in any of gRPC’s supported languages. So, for example, you can easily create a gRPC server in Java with clients in Go, Python, or Ruby. In addition, the latest Google APIs will have gRPC versions of their interfaces, letting you easily build Google functionality into your applications.
Performance:
- gRPC boasts speeds up to 10 times faster than REST APIs using JSON for communication. This is due to its use of Protocol Buffers, a lightweight and efficient binary format compared to text-based JSON.
Efficiency:
- Protobuf messages can be 30% smaller in size compared to JSON messages for the same data. This translates to less bandwidth usage and faster transmission times.
Scalability:
- gRPC leverages HTTP/2, which allows for efficient communication over a single connection. This is crucial for microservices architectures where numerous services communicate frequently.
Implementing gRPC in C# .NET: A Step-by-Step Tutorial
Setting Up Your Environment
Before we embark on the journey of implementing gRPC in C# .NET, it’s crucial to set up the development environment. Ensure you have the necessary tools and dependencies installed to kickstart your gRPC project seamlessly.
Defining Protobuf Messages
Protobuf, short for Protocol Buffers, is the language-agnostic data serialization format used by gRPC. Learn how to define your messages using Protobuf, providing a clear and efficient way for services to communicate.
Creating a gRPC Service
In this section, we’ll guide you through the process of creating a gRPC service in C# .NET. From defining the service contract to implementing the server logic, you’ll gain a comprehensive understanding of building robust services.
Implementing the gRPC Client
A well-rounded gRPC implementation involves both server and client components. Discover how to create a gRPC client in C# .NET, enabling seamless communication with your gRPC service.
Below is sample gRPC project create using visual studio 2019 and C#
Project Description : Request User ID and Return Customer Data C# gRPC Example
You can download full project here https://github.com/chandana-jagath/GrpcDemo.git
Server-Side Code
customers.proto
syntax = "proto3";
option csharp_namespace = "GrpcService";
service Customer{
rpc GetCustomerInfo(CustomerLockupModel) returns (CustomeModel);
}
message CustomerLockupModel{
int32 userId=1;
}
message CustomeModel
{
string firstName=1;
string lastName=2;
int32 age=3;
}
CustomerService.cs
using System.Threading.Tasks;
using Grpc.Core;
using Microsoft.Extensions.Logging;
public class CustomerService : Customer.CustomerBase
{
private readonly ILogger<CustomerService> _logger;
public CustomerService(ILogger<CustomerService> logger)
{
_logger = logger;
}
public override Task<CustomeModel> GetCustomerInfo(CustomerLockupModel request,
ServerCallContext context)
{
CustomeModel customeModel = new CustomeModel();
if (request.UserId == 1)
{
customeModel.FirstName = "AAA";
customeModel.LastName = "CCC";
customeModel.Age = 20;
}
if (request.UserId == 2)
{
customeModel.FirstName = "BBB";
customeModel.LastName = "DDD";
customeModel.Age = 30;
}
return Task.FromResult(customeModel);
}
}
Startup.cs
endpoints.MapGrpcService<CustomerService>();
Client-Side Code
Add Following Nuget Packages
- Google.Protobuf
- Grpc.Net.Client
- Grpc.Tools
static async Task Main(string[] args)
{
var input = new CustomerLockupModel { UserId = 5 };
var channel = GrpcChannel.ForAddress("https://localhost:5001");
var client = new Customer.CustomerClient(channel);
var reply = await client.GetCustomerInfoAsync(input);
Console.WriteLine($"{reply.FirstName} {reply.LastName} {reply.Age}");
Console.ReadLine();
}
Benefits of gRPC in C# .NET Development
Efficiency and Performance
One of the standout features of gRPC is its exceptional performance. By using HTTP/2 as the transport protocol and Protocol Buffers for serialization, gRPC minimizes latency and bandwidth usage, resulting in faster and more efficient communication.
Language Agnostic Communication
Thanks to its language-agnostic nature, gRPC enables communication between services implemented in different programming languages. This flexibility is a game-changer in microservices architectures where diverse technologies coexist.
Bidirectional Streaming and Multiplexing
Explore the power of bidirectional streaming and multiplexing in gRPC. These features allow for more complex communication patterns, making it easier to implement real-time applications and services.