Migrating PostgreSQL database: Comprehensive Guide
PostgreSQL, also known as Postgres, is a free and open-source relational database management system emphasizing extensibility and SQL compliance[https://en.wikipedia.org/wiki/PostgreSQL].In this article we are discussing how to Migrating PostgreSQL database in asp.net core with sample coding
Are you considering migrating your PostgreSQL database in ASP.NET Core? Look no further! In this guide, we’ll walk you through the process step by step, providing you with sample coding along the way. Whether you’re a seasoned developer or just starting out, this guide will help you seamlessly transition your database with confidence.
Contents
Understanding the Importance of Database Migration
Before diving into the migration process, it’s essential to understand why database migration is crucial for your ASP.NET Core application. By migrating your PostgreSQL database, you can take advantage of new features, improve performance, and ensure compatibility with the latest technologies. Additionally, migrating your database allows you to optimize its structure and enhance overall scalability, leading to a more efficient and robust application.
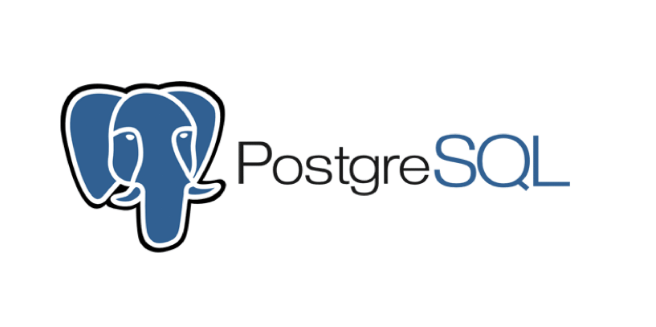
Preparing for Migration
The first step in migrating your PostgreSQL database is to back up your existing data. This ensures that you have a safe copy of your database in case anything goes wrong during the migration process. To back up your database, you can use tools like pg_dump or third-party backup solutions. Once you’ve backed up your data, you’re ready to proceed with the migration process confidently.
Setting Up Your ASP.NET Core Project
To begin migrating your PostgreSQL database, you’ll need to set up your ASP.NET Core project. If you haven’t already done so, create a new ASP.NET Core project or open an existing one in your preferred development environment. Make sure you have the necessary dependencies installed, including the Npgsql.EntityFrameworkCore.PostgreSQL package, which allows your ASP.NET Core application to communicate with PostgreSQL databases seamlessly.
1. Choosing your development environment:
There are multiple ways to create an ASP.NET Core project, but we’ll focus on using Visual Studio as it’s a popular choice for .NET development.
2. Fire up Visual Studio and create a new project:
- Open Visual Studio and navigate to “Create a new project”.
- In the “Create a new project” window, select “.NET Core” from the language filter and “Web” from the project type filter.
3. Selecting the project template:
- You’ll see various ASP.NET Core project templates. We’ll choose the most common one – “ASP.NET Core Web App (Model-View-Controller)”. This creates a project structure with pre-configured elements for building web applications using the Model-View-Controller (MVC) design pattern.
4. Naming your project:
- Provide a name for your project. For instance, let’s call it “MyBlogApp”.
5. Configuring additional options (optional):
- You can choose the target .NET version (e.g., .NET 7.0) based on your project requirements and compatibility needs.
- Uncheck the “Place solution and project in the same directory” option if you prefer a separate solution and project folder structure.
6. Hit “Create” and Visual Studio will set up your project:
This creates the core project files and folders. Here’s a breakdown of some important ones:
- Controllers folder: This will house the controller classes responsible for handling user interactions and requests.
- Models folder: This will contain your application’s data models representing entities like blog posts in our example.
- Views folder: This folder will hold the Razor view templates that define the user interface (UI) of your web pages.
Next steps:
From here, you can start building your web application! You can:
- Define models in the Models folder to represent your data. For example, a
BlogPost
model with properties like title, content, and author. - Create controllers in the Controllers folder to handle incoming requests and interact with models. A
BlogController
could handle displaying a list of blog posts or creating new ones. - Design views in the Views folder using Razor syntax to display content and user interfaces. A
Blog
view might display a list of blog posts retrieved from the controller.
Configuring Entity Framework Core for PostgreSQL
Once your project is set up, it’s time to configure Entity Framework Core to work with PostgreSQL. Start by adding a connection string to your appsettings.json file, specifying the necessary details such as the database server, port, username, and password. Next, register your DbContext in the ConfigureServices method of your Startup class, specifying Npgsql as the provider for PostgreSQL. With these configurations in place, Entity Framework Core is ready to interact with your PostgreSQL database effectively.
Connection String:
- Define a connection string in your application’s configuration file (e.g., appsettings.json) that specifies the details for connecting to your PostgreSQL database. Here’s an example connection string:
"ConnectionStrings": {
"DefaultConnection": "Host=your_postgres_server;Port=5432;Database=your_database;Username=your_username;Password=your_password;"
}
Data Context:
- Create a DbContext class that inherits from
DbContext
. This class represents the database context and will be used to interact with your PostgreSQL database. - In the
DbContext
constructor, use the connection string to configure the database provider.
- Entity Model:
- Define your data model using classes that represent your database tables. These classes will have properties that map to the columns in your tables.
- Use EF Core annotations (e.g.,
[Key]
,[Column]
) to configure the mapping between your classes and database tables.
- Migrations (Optional):
- If your database schema might change over time, it’s recommended to use EF Core Migrations. This allows you to define migration scripts that track changes to your data model and update the database schema accordingly.
- Use the
dotnet ef
command-line tools to generate and apply migrations.
- Working with Data:
- Once your EF Core configuration is complete, you can use the
DbContext
instance to interact with your PostgreSQL database. Here are some common operations:- Add new data: Use methods like
Add
orAddRange
on the relevantDbSet
property to add new entities to the database. - Retrieve data: Use methods like
Find
,FirstOrDefault
, orWhere
on theDbSet
to query and retrieve data from the database. - Update data: Load existing entities using
Find
or other methods, modify their properties, and callSaveChanges
on theDbContext
to persist the changes. - Delete data: Load existing entities and call
Remove
orRemoveRange
on theDbSet
to mark them for deletion. CallSaveChanges
to persist the deletion.
- Add new data: Use methods like
- Once your EF Core configuration is complete, you can use the
Generating Migrations
With Entity Framework Core configured, you can now generate migrations for your PostgreSQL database. Use the dotnet ef migrations add command to create a new migration, specifying a meaningful name that reflects the changes you’re making to your database schema. This command will generate a new migration file containing the necessary code to update your database schema accordingly.
Applying Migrations
Once you’ve generated your migrations, it’s time to apply them to your PostgreSQL database. Use the dotnet ef database update command to apply the pending migrations to your database, ensuring that your schema changes are reflected accurately. Entity Framework Core will automatically apply the necessary SQL scripts to migrate your database to the latest version, making the process seamless and efficient.
How to Migrating PostgreSQL database in asp.net core
Class File
public static IHost MigrateDatabase<TContext>(this IHost host, int? retry = 0) { int retryForAvailability = retry.Value; using (var scope = host.Services.CreateScope()) { var services = scope.ServiceProvider; var configuration = services.GetRequiredService<IConfiguration>(); var logger = services.GetRequiredService<ILogger<TContext>>(); try { logger.LogInformation("Migrating postresql database."); using var connection = new NpgsqlConnection (configuration.GetValue<string>("DatabaseSettings:ConnectionString")); connection.Open(); using var command = new NpgsqlCommand { Connection = connection }; command.CommandText = "DROP TABLE IF EXISTS Products"; command.ExecuteNonQuery(); command.CommandText = @"CREATE TABLE Products (Id SERIAL PRIMARY KEY, ProductName VARCHAR(24) NOT NULL, Description TEXT, Amount INT)"; command.ExecuteNonQuery(); command.CommandText = "INSERT INTO Products (ProductName, Description, Amount) VALUES('IPhone X', 'IPhone Discount', 150);"; command.ExecuteNonQuery(); command.ExecuteNonQuery(); logger.LogInformation("Migrated postresql database."); } catch (NpgsqlException ex) { logger.LogError(ex, "An error occurred while migrating the postresql database"); if (retryForAvailability < 50) { retryForAvailability++; System.Threading.Thread.Sleep(2000); MigrateDatabase<TContext>(host, retryForAvailability); } } } return host; }
Testing Your Migration
After applying your migrations, it’s essential to thoroughly test your PostgreSQL database to ensure that everything is working as expected. Write unit tests to validate your database schema changes and verify that your application functions correctly with the updated database structure. By testing rigorously, you can identify and address any potential issues early on, ensuring a smooth transition for your users.
Conclusion
In conclusion, migrating your PostgreSQL database in ASP.NET Core is a straightforward process that can yield significant benefits for your application. By following the steps outlined in this guide and leveraging sample coding provided, you can migrate your database with confidence and take advantage of the latest features and improvements offered by PostgreSQL. Remember to back up your data, configure Entity Framework Core correctly, generate and apply migrations, and thoroughly test your migration to ensure a successful outcome. With proper planning and execution, you can seamlessly transition your database and enhance the overall performance and scalability of your ASP.NET Core application.