Python Conditional Program: Free Guide
Introduction to Conditional Programming in Python
Python Conditional programming is a fundamental aspect of any programming language, including Python. It allows developers to create decision-making processes within their code, enabling the program to execute different sets of instructions based on certain conditions. Understanding conditional programming is crucial for writing efficient and functional Python code.
Contents
Basic Syntax of Conditional Statements in Python
In Python, conditional statements are primarily implemented using the if statement. The basic syntax of the if statement is as follows:
if condition:
# Code block to execute if condition is True
If the condition evaluates to True, the code block indented under the if statement is executed. Otherwise, it is skipped. Additionally, Python provides the if-else statement for branching execution based on whether the condition is True or False.
if condition:
# Code block to execute if condition is True
else:
# Code block to execute if condition is False
Nested if-else statements are also possible, allowing for multiple conditions to be evaluated sequentially.
Understanding Comparison Operators
Comparison operators are used to compare two values in Python. These operators include:
- Equal to (==)
- Not equal to (!=)
- Greater than (>)
- Less than (<)
- Greater than or equal to (>=)
- Less than or equal to (<=)
These operators return a Boolean value (True or False) based on the comparison result.
Logical Operators in Python
Python also supports logical operators, which are used to combine conditional statements. The logical operators include AND, OR, and NOT. These operators allow for the creation of complex conditions by combining multiple simpler conditions.
Ternary Operator in Python
Python also supports a ternary operator, which provides a concise way of writing conditional expressions. The syntax of the ternary operator is as follows:
variable = value_if_true if condition else value_if_false
Practical Examples of Python Conditional Programming
Let’s consider some practical examples to illustrate the usage of conditional programming in Python.
- Checking if a number is even or odd:
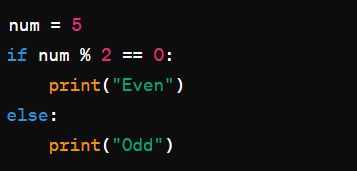
Best Practices for Writing Conditional Statements
When writing conditional statements in Python, it’s essential to follow best practices to ensure readability and maintainability of the code. These include:
- Using meaningful variable names and comments to explain the logic.
- Proper indentation to indicate the hierarchy of conditions.
- Avoiding deeply nested conditionals when possible to improve code clarity.
Error Handling with Conditional Statements
In addition to controlling the flow of execution, conditional statements can also be used for error handling. Python provides the try-except block for catching and handling exceptions gracefully.
try:
# Code that may raise an exception
except Exception as e:
# Code to handle the exception
Conclusion
In conclusion, conditional programming is a fundamental concept in Python that enables developers to create dynamic and responsive applications. By leveraging conditional statements, comparison operators, and logical expressions, programmers can implement complex decision-making processes within their code. Understanding the syntax and best practices of conditional programming is essential for writing clear, efficient, and maintainable Python code. Whether you’re a beginner learning the basics of programming or an experienced developer building sophisticated software systems, mastering conditional programming in Python is a valuable skill that opens up endless possibilities for innovation and creativity.
FAQs (Frequently Asked Questions)
- What is conditional programming? Conditional programming is a paradigm in which the execution of certain code blocks depends on specific conditions being met.
- Why is conditional programming important in Python? Conditional programming allows developers to create dynamic and flexible applications by enabling them to control the flow of execution based on varying conditions.
- What are some common mistakes to avoid when writing conditional statements in Python? Common mistakes include forgetting colons after if and else statements, misusing comparison operators, and not considering edge cases in the conditions.
- Can you provide an example of error handling with conditional statements in Python? Certainly! Error handling with conditional statements can be achieved using the try-except block, where the code that may raise an exception is placed within the try block, and the handling code is placed within the except block.
- How does conditional programming contribute to real-world applications? Conditional programming is vital in real-world applications across various domains such as web development, data analysis, and machine learning. It enables developers to create responsive and intelligent systems that adapt to changing conditions and user interactions.