Easy Way to Handling of ReflectionTypeLoadException in C#
Contents
Introduction
In the C# programming, developers often encounter the mysterious and challenging the handling of ReflectionTypeLoadException. Understanding how to effectively catch and manage this exception is crucial for creating robust and error-resistant applications. In this article, we delve into the depths of ReflectionTypeLoadException, providing insights and practical solutions to empower you in conquering this programming hurdle.
try
{
//The code that causes the error goes here.
}
catch (ReflectionTypeLoadException ex)
{
StringBuilder sb = new StringBuilder();
foreach (Exception exSub in ex.LoaderExceptions)
{
sb.AppendLine(exSub.Message);
if (exSub is FileNotFoundException)
{
FileNotFoundException exFileNotFound = exSub as FileNotFoundException;
if(!string.IsNullOrEmpty(exFileNotFound.FusionLog))
{
sb.AppendLine("Fusion Log:");
sb.AppendLine(exFileNotFound.FusionLog);
}
}
sb.AppendLine();
}
string errorMessage = sb.ToString();
//Display or log the error based on your application.
}
Demystifying Handling of ReflectionTypeLoadException
ReflectionTypeLoadException is a formidable challenge that developers face when dealing with reflection in C#. It occurs when there are issues loading types during reflection. This exception typically encapsulates multiple loader exceptions, making it a complex puzzle to solve. Let’s break down the key aspects and intricacies of ReflectionTypeLoadException.

The Culprits: Identifying Loader Exceptions
To effectively address ReflectionTypeLoadException, one must first identify the loader exceptions it encapsulates. These loader exceptions pinpoint specific issues encountered while loading types. Common culprits include missing assemblies, incompatible versions, or inaccessible dependencies. By unraveling these loader exceptions, developers gain valuable insights into the root causes of the exception.
Strategies for Exception Handling
Handling ReflectionTypeLoadException requires strategic approaches to ensure graceful degradation and robust application performance. Let’s explore effective strategies for catching and managing this exception.
1. Graceful Logging
Implementing a comprehensive logging mechanism is essential for capturing crucial information about ReflectionTypeLoadException instances. Logging helps developers track the occurrence of the exception, the associated loader exceptions, and relevant contextual data. This data proves invaluable in diagnosing and resolving issues promptly.
2. Targeted Exception Handling
Developers can employ targeted exception handling to address specific loader exceptions encapsulated within ReflectionTypeLoadException. This involves analyzing each loader exception type and applying tailored solutions. By taking a granular approach, developers can resolve issues systematically, ensuring a more resilient and fault-tolerant application.
3. Version Compatibility Checks
ReflectionTypeLoadException often arises from version conflicts between assemblies. Implementing version compatibility checks during the application’s initialization phase can preemptively identify and address potential issues. This proactive approach minimizes the likelihood of encountering ReflectionTypeLoadException in runtime scenarios.
Best Practices for Handling of ReflectionTypeLoadException-Free Code
Achieving a ReflectionTypeLoadException-free codebase requires adherence to best practices and proactive measures. Consider the following guidelines to enhance the resilience of your C# applications.
1. Maintain Up-to-Date Documentation
Keeping documentation current and comprehensive is instrumental in preventing ReflectionTypeLoadException. Accurate documentation aids in identifying dependencies, ensuring that the correct assemblies are referenced, and minimizing version conflicts.
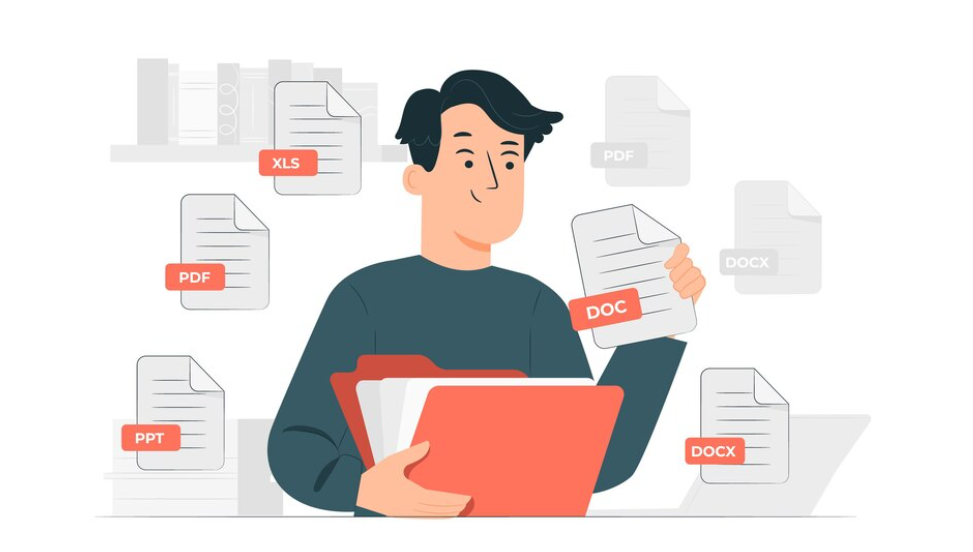
2. Regular Dependency Audits
Conducting regular audits of dependencies helps identify outdated or incompatible components. By staying vigilant and proactive in updating dependencies, developers can mitigate the risks of encountering ReflectionTypeLoadException in the long run.
3. Continuous Testing and Integration
Incorporating continuous testing and integration practices into your development workflow enables early detection of issues related to ReflectionTypeLoadException. Automated tests and continuous integration pipelines provide rapid feedback, allowing developers to address potential problems before they manifest in production environments.
Conclusion
ReflectionTypeLoadException may pose a formidable challenge, but with a deep understanding of its intricacies and strategic approaches to exception handling, developers can navigate this obstacle successfully. By implementing best practices, maintaining documentation, and embracing continuous improvement, you empower your C# applications to thrive in the dynamic world of software development.