Contents
20+ Advanced C# Interview Questions And Answers
If you’re aiming to a C# advanced interview, preparation is key. Beyond the basics, interviewers often search into advanced topics to assess your in-depth knowledge of C# programming. Here are the top 20+ advanced C# interview questions and answers along with detailed answers to help you shine in your next technical interview.
1. What is the purpose of the ‘yield’ keyword in C#?
In C#, ‘yield’ is used to create an iterator. It helps in the implementation of custom iteration patterns, allowing lazy loading of data, which enhances performance.
Answer: The ‘yield’ keyword in C# is utilized to create an iterator method. When used in a method, it indicates that the method will return an IEnumerable or IEnumerator collection. The ‘yield’ statement is employed to return each element one at a time, ensuring efficient memory usage.
public static IEnumerable<int> GenerateFibonacci(int count)
{
int a = 0, b = 1;
for (int i = 0; i < count; i++)
{
yield return a;
int temp = a;
a = b;
b = temp + b;
}
}
2. Explain the concept of covariance and contravariance in C#.
Covariance and contravariance enable implicit reference conversion for array types and delegate types. Covariance allows a more derived type to be used where a less derived type is expected, while contravariance allows the opposite.
Answer: Covariance in C# allows the use of a more derived type than originally specified, providing flexibility when working with arrays and delegates. Contravariance, on the other hand, permits the use of a less derived type, enhancing the reusability of code.
3. What are extension methods, and how do they differ from regular methods?
Extension methods in C# allow you to add new methods to existing types without modifying them. They are static methods that appear to be part of the original type, providing a convenient way to extend functionality.
Answer: Extension methods are static methods in a static class, and they are used to extend the functionality of existing types without altering their source code. Unlike regular methods, extension methods are called as if they were instance methods of the extended type.
public static class StringExtensions
{
public static bool IsUpperCase(this string str)
{
return str.Equals(str.ToUpper());
}
}
// Usage
bool isUpper = "HELLO".IsUpperCase(); // Returns true
4. Discuss the Singleton design pattern in C#.
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This pattern is useful when exactly one object is needed to coordinate actions across the system.
Answer: In C#, the Singleton design pattern involves creating a class with a method that creates a new instance of the class if one doesn’t exist. If an instance already exists, it returns the reference to that object. This guarantees a single point of access and avoids unnecessary instantiation.
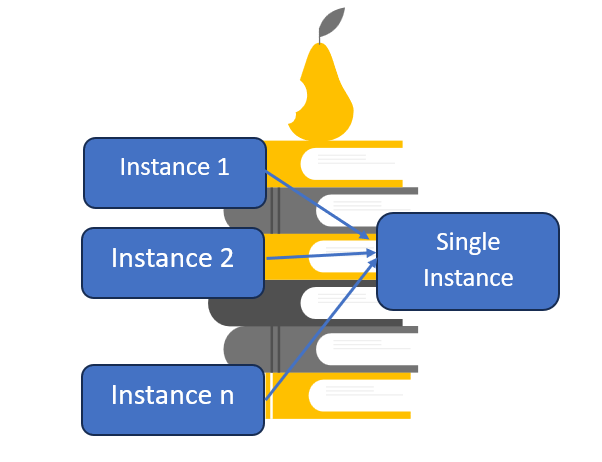
public class Singleton
{
private static Singleton instance;
private Singleton() { }
public static Singleton Instance
{
get
{
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
5. What is asynchronous programming in C#?
Asynchronous programming in C# allows you to perform non-blocking operations, enhancing the responsiveness of applications. The ‘async’ and ‘await’ keywords facilitate asynchronous programming by simplifying the syntax.
Answer: Asynchronous programming enables the execution of tasks concurrently without blocking the main thread. The ‘async’ keyword indicates that a method is asynchronous, while ‘await’ is used to await the completion of an asynchronous operation, preventing blocking and improving overall performance.
public async Task<string> ReadFileAsync(string filePath)
{
using (StreamReader reader = new StreamReader(filePath))
{
return await reader.ReadToEndAsync();
}
}
6. Explain the concept of garbage collection in C#.
Garbage collection in C# is an automatic memory management process. It identifies and collects objects that are no longer in use, freeing up memory and preventing memory leaks.
Answer: Garbage collection in C# automatically identifies and reclaims memory occupied by objects that are no longer reachable. The Common Language Runtime (CLR) handles garbage collection, ensuring efficient memory management and reducing the risk of memory-related issues.
7. Describe the use of the ‘using’ statement in C#.
The ‘using’ statement in C# is used for resource management, ensuring that the specified resources are properly disposed of when they are no longer needed.
Answer: The ‘using’ statement in C# is employed to define a scope within which a specified resource is utilized. Once the scope is exited, the ‘using’ statement ensures that the resource is disposed of, promoting efficient resource management and preventing resource leaks.
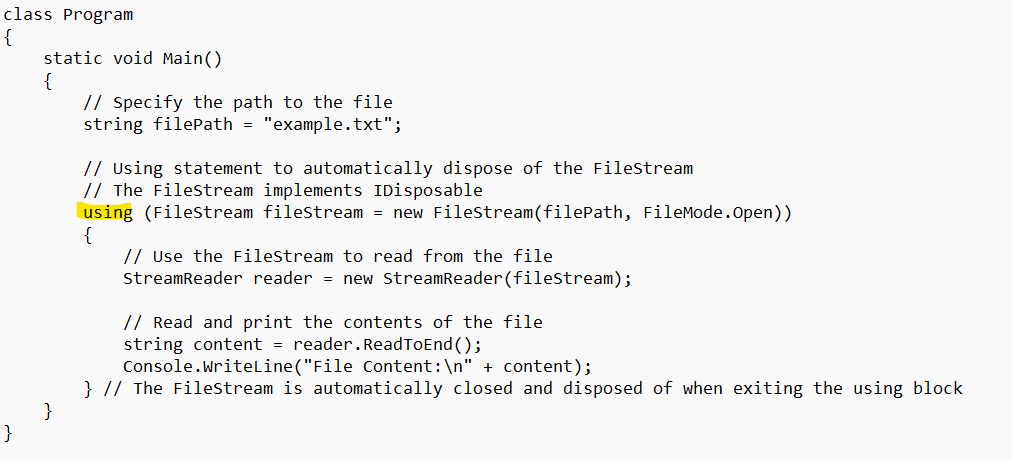
8. Elaborate on the difference between ‘readonly’ and ‘const’ variables in C#.
In C#, ‘readonly’ variables can only be assigned a value at the time of declaration or within the constructor, while ‘const’ variables are implicitly static and must be assigned a value at the time of declaration.
Answer: A ‘readonly’ variable in C# can be assigned a value either at the time of declaration or within the constructor of the containing class. In contrast, a ‘const’ variable must be assigned a value at the time of declaration and is implicitly static, making it a compile-time constant.
9. What is the purpose of the ‘out’ keyword in C#?
The ‘out’ keyword in C# is used to pass a variable by reference as an output parameter. It allows a method to return multiple values.
Answer: In C#, the ‘out’ keyword is employed in a method’s parameter list to indicate that the parameter is being passed by reference and is intended to be used as an output parameter. This enables the method to assign a value to the parameter, which can be accessed by the caller.
10. Explain the ‘async/await’ pattern in C#.
The ‘async/await’ pattern in C# simplifies asynchronous programming by allowing developers to write asynchronous code that resembles synchronous code, improving code readability and maintainability.
Answer: The ‘async/await’ pattern in C# is used to write asynchronous code in a more readable and synchronous-like manner. The ‘async’ keyword is applied to a method to indicate that it contains asynchronous code, while the ‘await’ keyword is used to asynchronously wait for the completion of a task.
11. Discuss the concept of delegates in C#.
Delegates in C# are type-safe function pointers that can reference methods with a specific signature. They enable the creation of callback mechanisms and are crucial for implementing events.
Answer: Delegates in C# are objects that refer to methods with a particular signature. They provide a way to encapsulate and pass methods as parameters, facilitating the implementation of callback mechanisms and events. Delegates play a vital role in achieving loose coupling in code.
12. How does C# support multiple inheritance?
C# does not support multiple inheritance through classes, but it supports it through interfaces. This allows a class to implement multiple interfaces, achieving a form of multiple inheritance.
Answer: C# avoids the complications of multiple inheritance through classes but supports it through interfaces. A class can implement multiple interfaces, enabling it to inherit behavior from multiple sources without the ambiguities associated with multiple inheritance through classes.
13. Discuss the concept of indexers in C#.
Indexers in C# provide a way to access elements in a class or struct using the array indexing syntax. They allow instances of a class to be treated like arrays.
Answer: Indexers in C# enable instances of a class or struct to be accessed using the same syntax as arrays. They provide a convenient way to encapsulate the internal representation of an object, allowing clients to access elements using indexers.
14. What is the purpose of the ‘using static’ directive in C#?
The ‘using static’ directive in C# simplifies code by allowing the use of static members of a class without specifying the class name.
Answer: The ‘using static’ directive in C# simplifies code by importing the static members of a class, enabling their use without specifying the class name. This enhances code readability and reduces verbosity when working with static members.
15. Explain the concept of the ‘partial’ keyword in C#.
The ‘partial’ keyword in C# allows a class, struct, interface, or method to be defined in multiple files. It facilitates the organization of large codebases by dividing the code into smaller, manageable parts.
Answer: The ‘partial’ keyword in C# is used to split the definition of a class, struct, interface, or method across multiple files. This feature is particularly useful for organizing large codebases and promoting better code management.
16. Discuss the role of attributes in C#.
Attributes in C# provide metadata about program entities, such as classes, methods, and properties. They enable additional information to be associated with code elements, facilitating enhanced reflection and code analysis.
Answer: Attributes in C# allow developers to attach metadata to program entities. This metadata can be used for various purposes, including enhancing reflection, code analysis, and providing additional information about the behavior of code elements.
17. What is the purpose of the ‘volatile’ keyword in C#?
The ‘volatile’ keyword in C# is used to indicate that a field can be accessed by multiple threads. It prevents certain compiler optimizations that might interfere with proper synchronization.
Answer: The ‘volatile’ keyword in C# is applied to fields to indicate that they can be accessed by multiple threads. It ensures that operations on the field are not optimized by the compiler in a way that could adversely affect the synchronization between threads.
18. Elaborate on the ‘ref’ and ‘out’ keywords in C#.
The ‘ref’ and ‘out’ keywords in C# are used for passing arguments by reference. While ‘ref’ is bidirectional, allowing input and output, ‘out’ is primarily used for output parameters.
Answer: The ‘ref’ keyword in C# is used for bidirectional parameter passing, allowing a method to modify the value of the parameter. The ‘out’ keyword, on the other hand, is specifically designed for output parameters, indicating that the method will assign a value to the parameter.
19. Discuss the concept of covariance and contravariance in generics.
Covariance and contravariance in generics allow for more flexibility when working with type parameters. Covariance permits the use of more derived types, while contravariance allows the use of less derived types.
Answer: Covariance in generics allows a more derived type to be used where a less derived type is expected, enhancing flexibility. Contravariance, on the other hand, permits the use of a less derived type, facilitating the reuse of code with different input types.
20. Explain the concept of the ‘using’ directive in C#.
The ‘using’ directive in C# is used to include a namespace in the program, allowing the use of types within that namespace without fully qualifying their names.
Answer: The ‘using’ directive in C# simplifies code by including a namespace and allowing the use of types within that namespace without specifying the fully qualified names. This enhances code readability and reduces verbosity when working with types from a specific namespace.
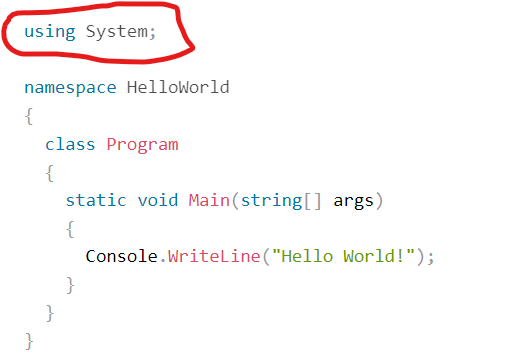
21. Discuss the benefits of using LINQ in C#.
Answer: LINQ (Language Integrated Query) allows querying various data sources using a consistent syntax, enhancing readability and reducing development time.
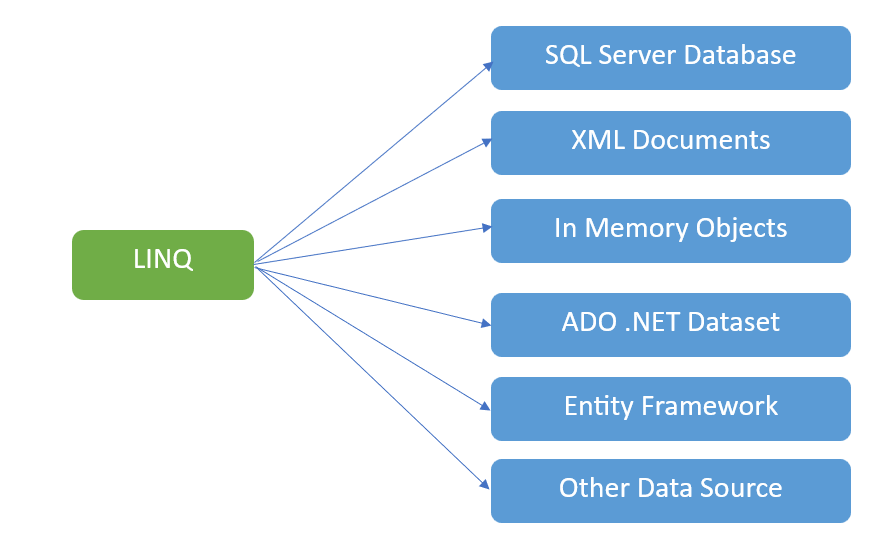
22. How does exception handling work in C#?
Answer: Exception handling uses ‘try,’ ‘catch,’ and ‘finally’ blocks to manage unexpected runtime errors, ensuring a graceful exit from unexpected situations.
try
{
// Code that might cause an exception
}
catch (ExceptionType ex)
{
// Handle the exception
}
finally
{
// Code that always executes, whether an exception occurred or not
}
finally
Block:
- The
finally
block is optional but is often used for code that must be executed regardless of whether an exception occurred. - It is useful for releasing resources like file handles or database connections.
In mastering these advanced C# interview questions and answers, you’ll not only demonstrate your proficiency but also gain a deeper understanding of the language’s intricacies. Remember to practice implementing these concepts in code to solidify your knowledge and approach your interview with confidence. Good luck!